Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial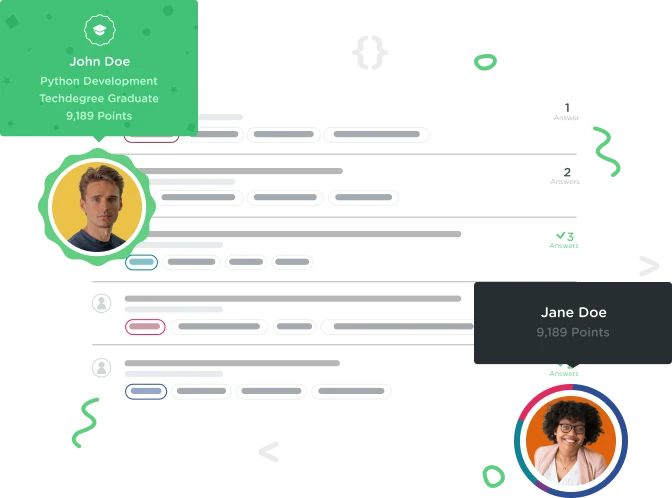
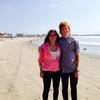
Taylor Plante
22,283 PointsWhy does Jason pass the argument (numbers=10) in the show_numbers method?
Around 2 minutes into this video, Jason begins writing an example of how to use blocks to count 0 through 9, but he doesn't exactly explain the first line of the method and I don't understand it:
def show_numbers(numbers=10)
i = 0
while i < 10
yield i
i += 1
end
end
show_numbers do |number|
puts "The current number is #{number}."
end
I tried changing the numbers=10 to numbers=8, numbers="string", and a few other things, and they all produced the same result. If you remove the =10 (or =anything), you get an error. Why is this necessary if the value is arbitrary? Is it arbitrary?
5 Answers
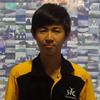
Sreng Hong
15,083 Pointsnumber = 10 is just the way to assign a default value of the parameter.
In this video, I think Jason forget to use this parameter in his function. So you can change the function look like this:
def show_numbers(numbers=10)
i = 0
while i < numbers #here
yield i
i += 1
end
end
show_numbers do |number|
puts "The current number is #{number}."
end
#you can call the function with the parameter too
show_numbers(5) do |number|
puts "The current number is #{number}."
end
Hope this helps.

Stone Preston
42,016 Pointsits been forever since ive done any ruby but if IIRC putting =someValue after a parameter name in a method assigns the parameter a default value, as to why its there or why it has to be there im not too sure.
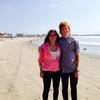
Taylor Plante
22,283 PointsThank you! I'm not sure if that's all there is to it, but sounds accurate. It was just way out of left field because every other block example he had done before he used the ampersand to tell ruby he was naming a block (i.e. &block), which I thought was necessary. Not the case?
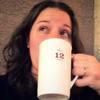
Ginger Bidwell
12,289 PointsI was wondering what the &block was for. As far as I understand, for the example given in this video, it isn't necessary because the method is not using the block as an object.
I wrote the example in the video as:
def get_name
print "Enter your name: "
name = gets.chomp
yield name
puts "It was nice to meet you, #{name}"
end
get_name {|name| puts "Hi, #{name.upcase}"}
Because I learned:
- You can do more complex things in interpolated strings besides just printing the value of the variable. In this case, the .upcase can be in there too.
- There's an informal best practice around when to use braces as opposed to do & end: if the precedence is not an issue, use braces for one-line blocks and do & end for mulitline blocks.
- Blocks are implicit parameters to the method - adding an ampersand on the last parameter makes this explicit, and turns the block into an object (of type Proc) which can be assigned to a variable and passed around.
I don't feel like I understand enough yet to write an example of a case for storing and then using a block, but I felt like I must have missed something because I didn't get what the &block was for and I didn't get what the numbers parameter was needed for here.
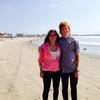
Taylor Plante
22,283 PointsThat makes perfect sense, thank you.
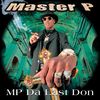
jay niceness
7,406 PointsSo can someone explain when or when the ampersand & is actually necessary ? very confused... thanks!

kelvin rodriguez
7,285 PointsFrom my understanding the main difference is that yield is not an object and thus is limited to the method that it is called in. & on the other hand can be passed to any method available to its class. For the most part yield is preferred.