Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial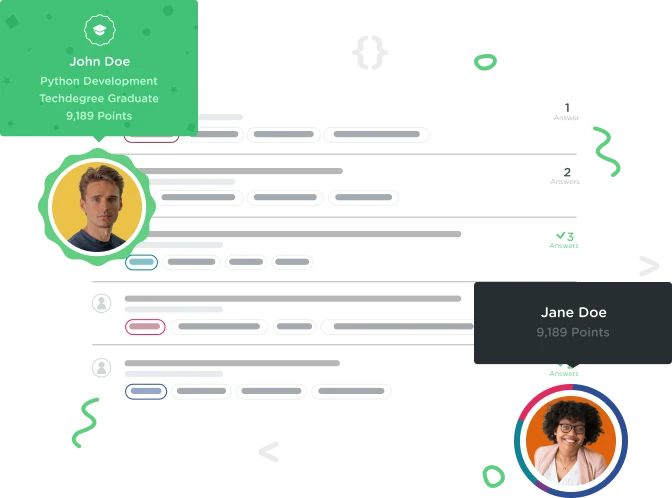
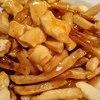
Routine Poutine
26,050 PointsWhy does JSX allow for strings without {}, but not numbers and booleans? "My Scoreboard" is OK, but not {5}.
Hi,
Why is it OK to use a "string" without {}, but not a number {5} or boolean {true}? Why aren't we required to put {"string"}?
3 Answers

Marco Fregoso
405 PointsYou can pass in the boolean or number value but since you're passing it directly it'll be parsed in as a string representation of that value. Let's say you passed the number 1 directly without curly braces.
const Container = () => {
return (
<div>
<Component passedValue="1" />
</div>
);
}
If you then tried to test what type of value the passed number is it would return a string which could cause annoying bugs if you tried to do something with the value.
const Component = (props) => {
console.log(typeof props.passedValue); // logs 'string' to the console
return ....
}
If on the other hand you were to pass the number in a JS expression with the value of 1 between curly braces it would be a number type.:
...
<Component passedValue={1} />
...
...
const Component = (props) => {
console.log(typeof props.passedValue); // logs 'number' to the console
return ....
}
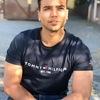
MD MONIRUZZAMAN
6,130 PointsThis { }
Curly braces lets JSX know that it's a javascript expression & need to evaluate. Like isFun={true}
.
When we use { }
in JSX ,it's called a JSX expression
<Header
title="My Scoreboard"
totalPlayers={5}
isFun={true}
/>
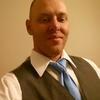
David Turner
1,061 PointsGreat question. My understanding is that as the JSX mimics the name/value attribute system from HTML, you must pass it either a string like you would in HTML <a href="www.google.com"></a>
(the href
portion of that tag is the attribute) or to delineate a different method, you must use the expression evaluator {}
.
As someone mentioned above, passing a number in quotes would evaluate to a string and you would have to do type conversion or check the string value to use it as a number (not very handy or fun).