Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial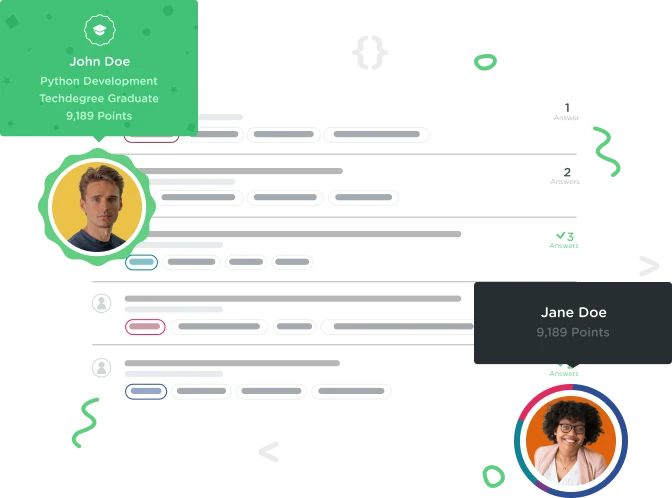

Derek Markman
16,291 PointsWhy does my activity_main.xml include extra layout code?
Here's my activity_main.xml file:
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/main_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
tools:context=".MainActivity">
<android.support.design.widget.AppBarLayout
android:id="@+id/appbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingTop="@dimen/appbar_padding_top"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/AppTheme.PopupOverlay"
app:layout_scrollFlags="scroll|enterAlways">
</android.support.v7.widget.Toolbar>
<android.support.design.widget.TabLayout
android:id="@+id/tabs"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</android.support.design.widget.AppBarLayout>
<android.support.v4.view.ViewPager
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"/>
</android.support.design.widget.CoordinatorLayout>
Do I need to change this at all to do these lessons?
2 Answers

Derek Markman
16,291 PointsI have already done that and tried changing it. But it throws a ton of errors at me when I change it to what Ben has it with just the relative layout. And yes I am on AS 1.4

Ben Deitch
Treehouse TeacherAre you using the right layout file? Ben's activity_main uses a ViewPager. What errors was it showing?

Derek Markman
16,291 PointsI'm currently not home so I can't take a look at the code. It's possible I have something confused here I'll reply back once I'm able to look at all of the code later today. Thanks for the replys

Derek Markman
16,291 PointsWell my errors come from my MainActivity.java file where it cant find the symbol variables specified inside the activity_main.xml file. The symbol variables it can't find specifically are: variable toolbar, variable container, variable tabs.
Here's my MainActivity.java file
package com.teamtreehouse.ribbit;
import android.content.Intent;
import android.os.Bundle;
import android.support.design.widget.TabLayout;
import android.support.v4.app.FragmentPagerAdapter;
import android.support.v4.view.ViewPager;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import com.parse.ParseAnalytics;
import com.parse.ParseUser;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link FragmentPagerAdapter} derivative, which will keep every
* loaded fragment in memory. If this becomes too memory intensive, it
* may be best to switch to a
* {@link android.support.v4.app.FragmentStatePagerAdapter}.
*/
private SectionsPagerAdapter mSectionsPagerAdapter;
/**
* The {@link ViewPager} that will host the section contents.
*/
private ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ParseAnalytics.trackAppOpenedInBackground(getIntent());
ParseUser currentUser = ParseUser.getCurrentUser();
if (currentUser == null) {
navigateToLogin();
}
else {
Log.i(TAG, currentUser.getUsername());
}
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(this,
getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.container);
mViewPager.setAdapter(mSectionsPagerAdapter);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tabs);
tabLayout.setupWithViewPager(mViewPager);
}
private void navigateToLogin() {
Intent intent = new Intent(this, LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_logout) {
ParseUser.logOut();
navigateToLogin();
}
return super.onOptionsItemSelected(item);
}
}

Derek Markman
16,291 PointsThose symbol variables are specified in lines:
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
mViewPager = (ViewPager) findViewById(R.id.container);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tabs);
And if you take another look at my activity_main.xml file you'll see where it's calling each of those symbol variables.

Ben Deitch
Treehouse TeacherLooks like the new project templates are starting to complicate things. Ben J doesn't have a toolbar or tablayout variable, and the ID for mViewPager is R.id.pager. Also, it looks like old project template would generate a lot more methods at the bottom of the class to help with tabs. I'd try comparing you're MainActivity to Ben's and adding anything you're missing and getting rid of anything extra. If that doesn't work, maybe post your project to Github?

Derek Markman
16,291 PointsNah i don't think its worth posting to github, a lot of my code doesn't match up to bens but no worries I've got everything working currently so I'll just try to adapt to these super out of date videos
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherI'd change it to be safe. Are you on Android Studio version 1.4? It changed the default project templates :(. You can check the project files in the Downloads section of the video if you want to see what Ben J's file looks like.