Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial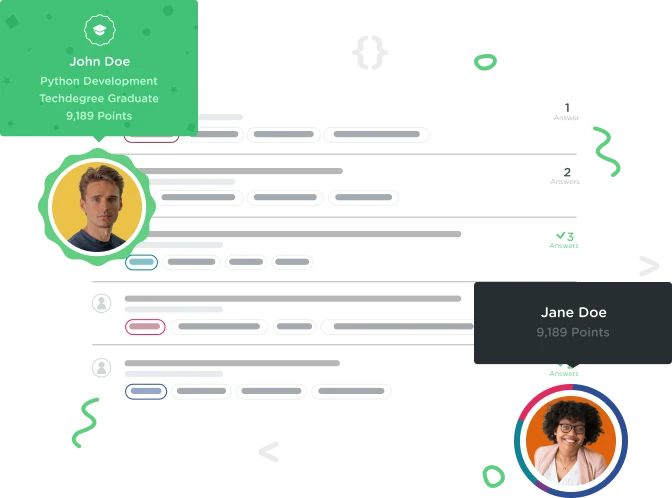

Daniel Hardeman
1,089 PointsWhy does my alert dialog not trigger when the user enters a non-number value?
Here is my code:
/**
- Returns a random number between 2 user-defined numbers
- @param {number} lower - the lowest number value.
- @param {number} upper - the highest number value.
- @return {number} The random number value. */
function getRandomNumber( lower, upper )
{
const lowerInput = prompt(Enter a minimum number:
);
const upperInput = prompt(Enter a maximum number:
);
lower = parseFloat(lowerInput);
upper = parseFloat(upperInput);
const main = document.querySelector('main');
const randomNumberGen = Math.floor(Math.random() * ( upper - lower + 1)) + lower;
if ( lower !== isNaN(lower) || upper !== isNaN(upper))
{
return main.innerHTML = `<h1>Random Number Generator:</h1>
<h3>Choose a minimum and maximum number and the Random Number Generator will randomly select a number between the two.</h3>
<p>${randomNumberGen} is a random number between ${lower} and ${upper}.</p>`;
}
else
{
alert('You must enter two numbers. Please reload and try again.')
}
}
getRandomNumber();
3 Answers
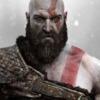
boi
14,242 PointsYour conditional statement (if ()
) is wrong.
Your conditional statement is not doing what you might expect, instead what it's actually doing is this:
If a string
is passed.
if ( NaN !== true || NaN !== true)
If a number
is passed say 5
and 10
.
if ( 5 !== false || 10 !== false )
In both cases, the if
condition is coming out TRUE. So in reality the else
never gets executed.

Michael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 Pointslet lower = Nan;
if (lower !== isNaN(lower))
If lower is a Nan, the if equates to if( Nan !== true ) so the if executes because it's true, Nan is not equal to true.

Anna Silvester
Full Stack JavaScript Techdegree Student 3,870 PointsWould this work?
If either upper or lower are not numbers then the condition is true. Otherwise they are both numbers and the else statement will execute.
The value of isNaN(lower) is either true or false so can be written this way.
if ( isNaN(lower) || isNaN(upper))
{
alert('You must enter two numbers. Please reload and try again.')
}
else
{
return main.innerHTML = `<h1>Random Number Generator:</h1>
<h3>Choose a minimum and maximum number and the Random Number Generator will randomly select a number between the two.</h3>
<p>${randomNumberGen} is a random number between ${lower} and ${upper}.</p>`;
}