Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial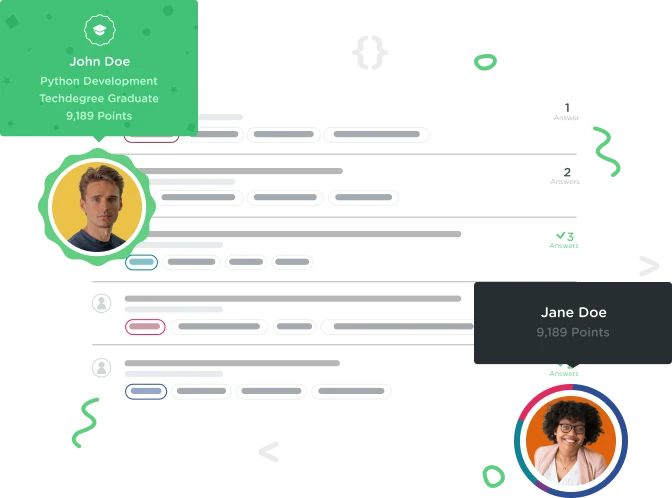

Rob Morris
1,968 PointsWhy does my code work with document.write but not the document.getElementByID?
Please see my print function -
- This worked when using the classic document.write method
- however, this did not work when using the tutorial-suggested getElementById
why is this?
Also. please comment on my coding so I know if I've over done it or not. Thanks in advanced.
var questions = [
['How many legs does a cat have?', 4],
['What is my age?', 26],
['how many years of driving?', 1]
];
var correctAnswers = 0;
var question;
var answer;
var response;
var htmlCorrectAnswers;
var questionsCorrect = [];
var questionsIncorrect = [];
function print(message) {
// this works correctly
document.write(message)
// this method does not work correctly
// var outputDiv = document.getElementById('output');
// outputDiv.innerHTML = message; // this method does not work correctly
}
//This for loop compares your answer and push's the question to the appropriate variable depending on whether it was correct or incorrect
for (i = 0; i < questions.length; i += 1){
question = questions[i][0];
answer = questions[i][1];
response = parseInt(prompt(question));
if (answer == response){
questionsCorrect.push(question)
correctAnswers += 1
} else {
questionsIncorrect.push(question)
}
}
htmlCorrectAnswers = '<b><p>You got ' + correctAnswers + ' question(s) right</b></p>';
// This is a function that prints correctly answered questions
function printCorrectAnswers (list){
if(questionsCorrect == 0){
print('<p><b>You did not get an answer correct ahahah! </b></p>')
} else {
var listHTML = '<ol><b>You managed to get the following questions right:</b>';
for (i = 0; i < questionsCorrect.length; i += 1 ){
listHTML += '<li> ' + list[i] + ' </li>';
}
listHTML += '</ol>';
print(listHTML);
}
}
// This is a function that prints incorrectly answered questions
function printIncorrectAnswers (list){
if(questionsIncorrect == 0){
print('</p><b>You did not get an answer wrong</b></p>')
} else {
var listHTML = '<ol><b>You got the following questions wrong:</b>';
i = 0;
for (i = 0; i < questionsIncorrect.length; i += 1){
listHTML += '<li> ' + list[i] + ' </li>';
}
listHTML += '</ol>';
print(listHTML);
}
}
print(htmlCorrectAnswers);
printCorrectAnswers(questionsCorrect);
printIncorrectAnswers(questionsIncorrect);
Please
2 Answers
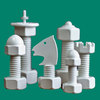
Steven Parker
231,846 PointsThe method of using document.write will place content on a completely empty page.
The other method relies on the page already having a div
element on it with an ID property with the value "output". If that element does not exist on the page to begin with, nothing will be seen.
Example starting page contents:
<div id="output"></div>
UPDATE: The document.write function appends to the page, so each time you use it, you add to what was written already.
But "outputDiv.innerHTML = message
" replaces the content of the div each time it is executed. So you'll only see what was written the last time it was done.
You can make it work more like the original using the concatenating assignment operator:
outputDiv.innerHTML += message;

Rob Morris
1,968 PointsThanks, Steven, your last answer here makes perfect sense & works perfect - Can't believe I missed it. Shame I can't actually select that response as the "best answer"
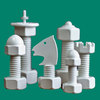
Steven Parker
231,846 PointsI've updated my original answer and removed the comment.
Rob Morris
1,968 PointsRob Morris
1,968 PointsSorry, I'll have to rephrase my point.
My HTML does have the output ID & the first function runs OK, which displays how many of each I got right. The other functions which are called do not display which was right or wrong.