Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial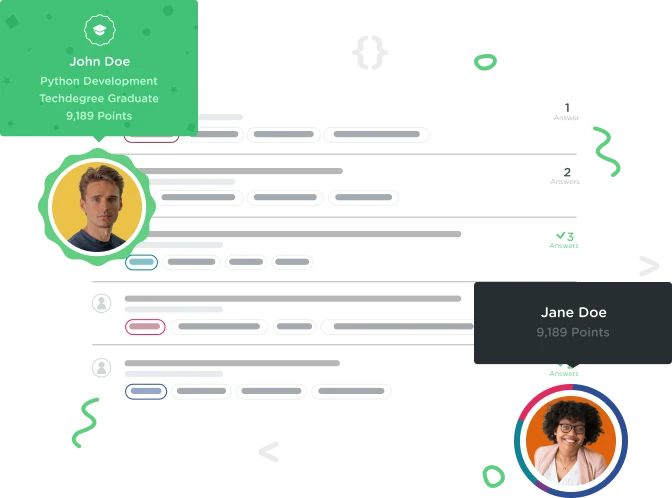

Braeden Long
2,137 PointsWhy does my do..while loop not repeat the prompt?
When I tried to complete the challenge I ended up writing a do... while loop instead of a while loop. Here is my version.
do {
search = prompt("Search for a student's records by name [i.e. Dave]. If you wish to view the results, type quit.")
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search === student.name) {
message = getStudentReport(student)
print(message);
}
}
} while (search === null || search.toLowerCase === 'quit')
Here is the instructor's.
while (true) {
search = prompt("Search for a student's records by name [i.e. Dave]. If you wish to view the results, type quit.")
if (search === null || search.toLowerCase === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search === student.name) {
message = getStudentReport(student)
print(message);
}
}
}
I'm curious to know what makes the teacher's loop continuously prompt the user. The user MUST type quit or cancel to exit the continuous prompts, but with my do... while loop I can type anything and the prompt will only execute that one time. What is the difference between the two loops? I set my condition so that the loop should end when the user inputs 'quit' or cancels the prompt, so in theory, the prompt should pop up continuously, correct? Why is it not, then?
2 Answers

Shadab Khan
5,470 PointsHi Braeden,
It's an excellent question. Here's the solution to your problem:
First, toLowerCase is a JavaScript function, so it must be always followed by a parenthesis like so: examplestring.toLowerCase();
Secondly, you need to exit the loop in case when the user enters "quit" or closes the application, which you have implemented as the following condition : while (search === null || search.toLowerCase === 'quit') , if you notice carefully, this condition evaluates to true whenever you enter quit or close the application and will keep the loop going.
What you need to do is 'negate' the above condition; which is like so, while (!(search === null || search.toLowerCase() === 'quit')), which basically means that keep the do..while loop going until the user doesn't enter quit or chooses to close the application
Here is how the final script should be like:
do
{
search = prompt("Search for a student's records by name [i.e. Dave]. If you wish to view the results, type quit.");
for (var i = 0; i < students.length; i += 1)
{
student = students[i];
if (search === student.name)
{
message = getStudentReport(student)
print(message);
}
}
} while (!(search === null || search.toLowerCase() === "quit"));
Also, there is a difference between the instructor's code and yours. In the isntructor's case, he has decided to quit the loop using 'break' statement when the user enters quit or closes the application, but in your case you have done vice-versa, which is you've basically instructed the code to keep going if you see carefully :)
Hope that helps, let me know if you have any further questions. All the best.

Shadab Khan
5,470 PointsHi Braeden,
There's one real difference between the two, which must be applied on a requirement basis:
The 'while' loop will not even execute once if its condition fails, on the other hand, the do...while loop will execute atleast once before checking its looping condition to decide if it needs to continue looping or break out of it.
So to summarise, if you wish to execute the block of code 'atleast' once regardless of the condition, then use do...while, else use the while loop.
All the best!
Braeden Long
2,137 PointsBraeden Long
2,137 PointsThanks for your help. It all came down to how I understood the process. I was thinking that it executed the code UNTIL the condition was met, but actually, it executes the code WHILE the condition holds true. Thank you so much for the help.
Would there be any reason to use the do loop instead of the do...while loop in this particular instance? It seems to just be a matter of preference.