Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial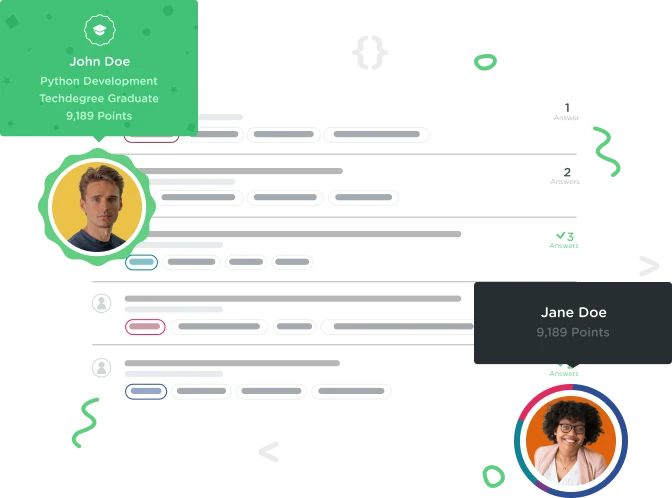

Kylie Nonemaker
1,190 PointsWhy does my grid have a period as a placeholder?
My code is:
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat', 'Dev', 'Egg', 'Far', 'Gum', 'Hut']
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append('. ')
return row
def create_grid(self):
# / A / B / C / D /
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row) + ' |'
print(print_row)
# methods
# create our grid
# check for matches
# check game won
# run the game
# dunder main
if __name__ == '__main__':
game = Game()
game.set_cards()
game.cards[0].matched = True
game.cards[1].matched = True
game.cards[2].matched = True
game.cards[3].matched = True
game.create_grid()
# call start game
The result I get is:
treehouse:~/workspace$ python game.py
| A | B | C | D |
1| . | . | . | . |
2| . | . | . | . |
3| . | . | . | . |
4| . | . | . | . |
treehouse:~/workspace$ python game.py
| A | B | C | D |
1| . | . | . | . |
2| . | . | Add | Add |
3| . | . | . | Boo |
4| . | Boo | . | . |
In the first run, I didn't add the match attributes. In the second run, I added the matched attributes to see if spacing would be off - but it looks like it's a placeholder but I can't find where in my code that is. I was following along with the video so I think there must be a typo as it wasn't intentional.
1 Answer

jb30
44,807 Points def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append('. ')
return row
Try changing row.append('. ')
to row.append(' ')