Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial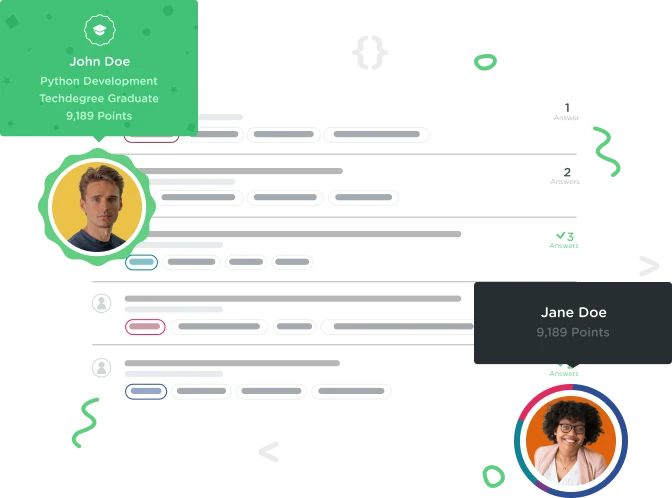

diginoma
Courses Plus Student 8,009 PointsWhy does my Grid print this way?
| A | B | C | D |
1| | | |
2| | | |
3| | | |
4| | | |
The lines in the final column are missing...

diginoma
Courses Plus Student 8,009 Pointsfrom cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['add', 'boo', 'cat', 'dev',
'egg', 'far', 'gum', 'hut']
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
def create_grid(self):
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row)
print(print_row)
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for car in cards:
print(f'{card.location}: {card}')
return False
def check_win(self):
for card in self.cards:
if card.matched == False:
return False
else:
return True
def check_location(self,time):
while True:
guess = input(f"What's the location of your {time} card? ")
if guess.upper() in self.locations:
return guess.upper()
else:
print("That's not a valid location")
def start_game(self):
game_running = True
print('Memory Game')
self.set_cards()
while game_running:
self.create_grid()
guess1 = self.check_location('first')
guess2 = self.check_location('second')
if self.check_match(guess1, guess2):
if self.check_win():
print('congrats! You have guessed them all!')
self.create_grid()
game_running = False
else:
input('Those cards are not a match.Please try again')
print('Game Over')
if __name__ == '__main__':
game = Game()
game.start_game()
game.set_cards()
game.create_grid()

diginoma
Courses Plus Student 8,009 Pointsclass Card:
def __init__(self, word, location):
self.card = word
self.location = location
self.matched = False
def __eq__(self, other):
return self.card == other.card
def __str__(self):
return self.card
if __name__== '__main__':
card1 = Card('egg', 'A1')
card2 = Card('egg', 'B1')
card3 = Card('hut', 'd4')
print(card1 == card2)
print(card1 == card3)
print(card1)

diginoma
Courses Plus Student 8,009 PointsThanks in advance!
1 Answer

jb30
44,806 Points def create_grid(self):
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row)
print(print_row)
Have you tried changing print_row += ' | '.join(get_row)
to print_row += ' | '.join(get_row) + ' |'
?

diginoma
Courses Plus Student 8,009 Points-facepalms- thanks bro
jb30
44,806 Pointsjb30
44,806 PointsCan you post your code related to printing your Grid?