Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial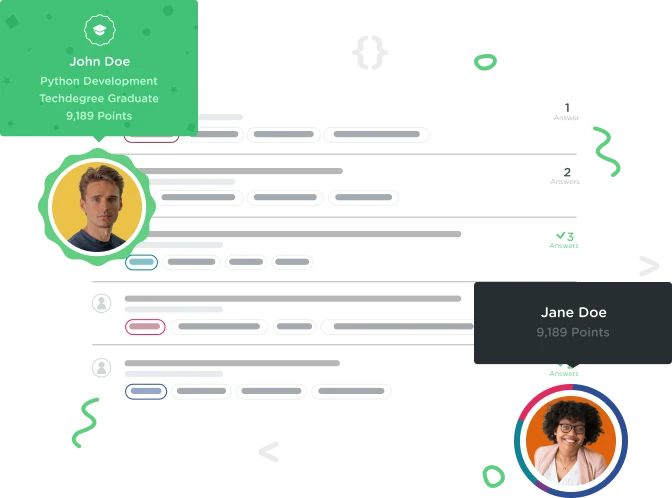

Payton Roedel
1,555 PointsWhy does my program break when I add a 'QUIT' option to exit application?
When new_item == 'DONE', my program works just fine, but why does it completely break when I change that to new_item == 'DONE' or 'QUIT'?
The problem happens on line 41: if new_item.upper() == 'DONE' or 'QUIT':
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name =="nt" else "clear")
def num_items(shopping_list):
print ("List has {} items.".format(len(shopping_list)))
def add_to_list(item):
item = item.upper()
shopping_list.append(item)
print ("Added!")
show_list(shopping_list)
def remove_item(item):
item = item.upper()
shopping_list.remove(item)
def show_help():
clear_screen()
print("What should we get at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' to show this menu.
Enter 'SHOW' to show list.
Enter 'REMOVE' to remove items
""")
def show_list(shopping_list):
print ("List has {} items.".format(len(shopping_list)))
for item in shopping_list:
print("* " + item)
#THIS IS THE LOGIC
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE' or 'QUIT':
show_list(shopping_list)
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list(shopping_list)
continue
elif new_item.upper() == 'REMOVE':
item = input("Which item would you like to remove? ")
remove_item(item)
show_list(shopping_list)
else:
add_to_list(new_item)
```
2 Answers
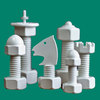
Steven Parker
230,274 PointsAs Jasper's example shows, you need to use complete expressions on both sides of the logic "or" operation. A non-empty string on one side by itself will be considered "truthy".
A more compact way of testing for multiple answers would use the membership operator ("in") with a list:
if new_item.upper() in ['DONE', 'QUIT']:

Payton Roedel
1,555 PointsOk that makes sense now. It wasn't giving an error so I couldn't follow the logic very easily (still pretty new). Are you able to explain why "if new_item.upper() == 'DONE' or 'QUIT':" doesn't work logically?
The line you give Steven looks simple yet effective and I will for sure be using the format in future code.
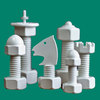
Steven Parker
230,274 PointsSo a plain string (no comparison) is "truthy", so you can replace it with "True". Then anything "or"-ed with True will still be True. So:
if new_item.upper() == 'DONE' or 'QUIT': # this is the same thing as....
if (new_item.upper() == 'DONE') or ('QUIT'): # which is the same as...
if new_item.upper() == 'DONE' or True: # which is the same as...
if True:
Does that explain it? Happy coding!

Payton Roedel
1,555 PointsThank you Jasper as well, I don't know how my eyes didn't read the "or new_item.upper() == 'QUIT':"
That's what you get when you are new to coding and try to type while listening.
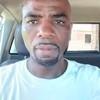
Jasper Maposa
26,918 Points@Payton. It happens to us all, we are ever learning. Glad that you now have a deeper understanding of what you have asked.
Jasper Maposa
26,918 PointsJasper Maposa
26,918 PointsI think the correct syntax is
if new_item.upper() == 'DONE' or new_item.upper() == 'QUIT':