Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial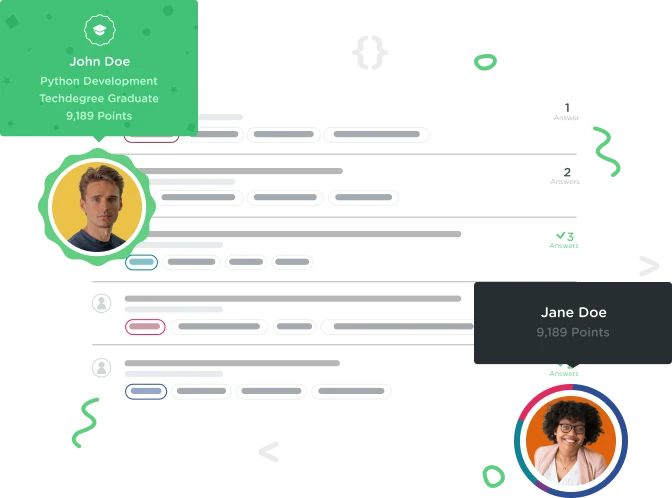

Jackson Monk
4,527 PointsWhy does next work, but previous doesn't?
I have a couple of lists I cycle through here. For each category, one is full of the divs that qualify for that category, and the other is full of each div's padding element. The padding elements are just to space the div from the next list. I have two categories. likes and comments, and cycle through the lists for each of them using a next and previous button for each, like this:
next[0].addEventListener('click', (event) => {
for (let y = 0; y < firstList.children.length; y++) {
if (!likeRantDivs[y + 1]) break;
if (likeRantDivs[y].style.display != 'none') {
$(likeRantDivs[y]).fadeOut(400);
$(likeRantFillers[y]).fadeOut(450);
$(likeRantDivs[y + 1]).fadeIn(400);
$(likeRantFillers[y + 1]).fadeIn(350);
break;
}
}
});
prev[0].addEventListener('click', (event) => {
for (let z = 0; z < firstList.children.length; z++) {
if (!likeRantDivs[z - 1]) break;
if (likeRantDivs[z].style.display != 'none') {
$(likeRantDivs[z]).fadeOut(400);
$(likeRantFillers[z]).fadeOut(450);
$(likeRantDivs[z - 1]).fadeIn(400);
$(likeRantFillers[z - 1]).fadeIn(350);
break;
}
}
});
next[1].addEventListener('click', (event) => {
for (let zy = 0; zy < secondList.children.length; zy++) {
if (!commentRantDivs[zy + 1]) break;
if (commentRantDivs[zy].style.display != 'none') {
$(commentRantDivs[zy]).fadeOut(400);
$(commentRantFillers[zy]).fadeOut(450);
$(commentRantDivs[zy + 1]).fadeIn(400);
$(commentRantFillers[zy + 1]).fadeIn(350);
break;
}
}
});
prev[1].addEventListener('click', (event) => {
for (let zz = 0; zz < secondList.children.length; zz++) {
if (!commentRantDivs[zz - 1]) break;
if (commentRantDivs[zz].style.display != 'none') {
$(commentRantDivs[zz]).fadeOut(400);
$(commentRantFillers[zz]).fadeOut(450);
$(commentRantDivs[zz - 1]).fadeIn(400);
$(commentRantFillers[zz - 1]).fadeIn(350);
break;
}
}
});
As you can see, I have next and previous buttons for both the likes and comments lists. Each time the for loop runs for each of these event listeners, it basically says if the current div is not hidden, meaning it is the one currently visible, then fade out the current one and fade in the next one. That part works fine, but it's that first if statement right after each of the for loops that is the trouble. if (!likeorcommentRantDivs[loopVariable -or+ 1]) break;
This tries to say that if there is no next or previous div, meaning the current one is the last or first in the list, then break, and just leave the current one be. This is where the problem occurs, because this line works on both of the next statements, but on neither of the previous ones, even though I changed their brackets to read [var - 1] instead of [var + 1]. Why would this be?
2 Answers
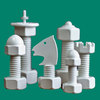
Steven Parker
231,275 PointsIn both cases (next and prev) the calculated index will cause an out-of-bounds reference, and you have a check for a non-existent reference that will end the loop.
The obvious difference is that with "next", it will not occur until the last step of the loop, but with "prev" it will occur on the very first one so no actions will be performed.
Instead of the test and abort (break), you could just constrain the loop so the indexes end before the last item for "next", and start at 1 instead of 0 for "prev".

Jackson Monk
4,527 PointsAh, that does make sense Steven, but I think I forgot to mention that when I hit next a couple times and go farther into the list of divs, then hit previous, it still doesn't do anything. If I were four or five items in, wouldn't that if statement be false when I hit previous?
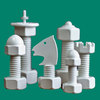
Steven Parker
231,275 PointsIt doesn't matter where you click, the "event" is passed to the handler, but it never uses it. Instead, it always loops through each index starting from the beginning (0), and the first thing it does is check for the existence of the item at the previous index.