Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial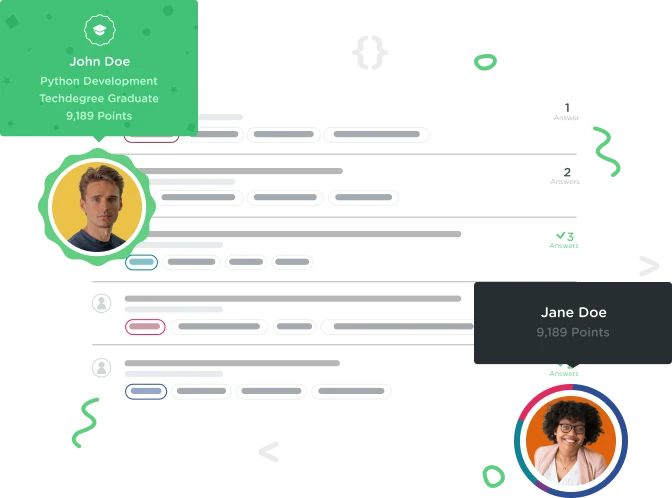

xajx
6,553 PointsWhy does nums.index(10) show a ValueError: 10 is not in list?
I'm very confused with this.
nums = range(1, 10, 2)
nums.index(5) # 2 nums.index(10) # ValueError: 10 is not in list nums.index(1) # 0
Why does nums.index(5) show a 2 when it's not in the tuple? Why does nums.index(10) show a value error?
2 Answers
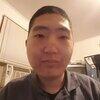
Joseph Yhu
PHP Development Techdegree Graduate 48,638 Points- You are starting at 1 and incrementing by 2, so only the odd numbers would be returned. Also the stop value in the range function isn't included anyway.
- Remember the indices start at 0, so nums[0] = 1, nums[1] = 3, and nums[2] = 5.

ygh5254e69hy5h545uj56592yh5j94595682hy95
7,934 Points# this does not really create a list
nums = range(1, 10, 2)
# if you print nums
# you will get
range(1, 10, 2)
# if you want to get a list from range(1, 10, 2), you will need to wrap this in a list(), like so
nums = list(range(1, 10, 2))
# ^ this will now return this when you try to print nums:
[1, 3, 5, 7, 9]
# ^ this makes sense, since range says to start at 1, skip every 2, until 10
# so all odd numbers from 1 - 10, which are 1, 3, 5, 7, 9
# Now, the reason why you where getting 2 when you typed:
nums.index(5)
# is because if you look at the list, [1, 3, 5, 7, 9]
# the number 5 is in the 2 index of that list
# so what .index(X) does, it look at the list for whatever number is passed in
# and it returns the index where that number is located
# The reason why you were getting ValueError when you typed:
nums.index(10)
# is because 10 is not in the list [1, 3, 5, 7, 9]
# if you did
nums.index(1) # you would get 0
nums.index(3) # you would get 1
nums.index(5) # you would get 2
nums.index(7) # you would get 3
nums.index(9) # you would get 4
# hope that helps