Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial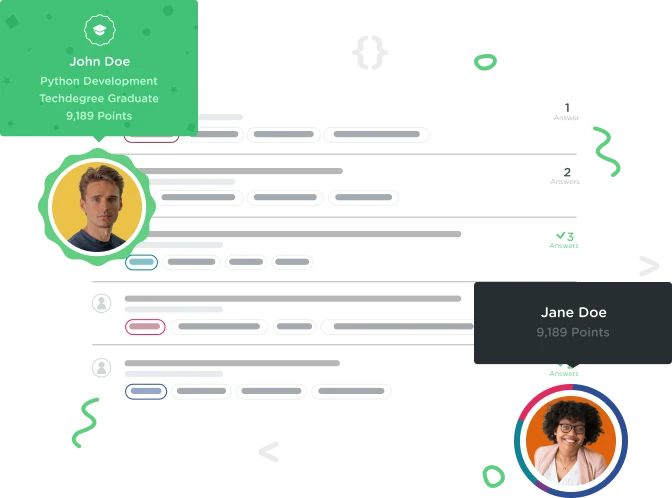
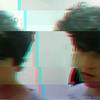
Daniel Maia
2,559 PointsWhy does object.onmouseover is instantly triggered with no actual mouse over?
Ok, so I've been trying some experiments with javascript. Here's my HTML:
<html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title> Classy </title> <script type="text/javascript" src="javascript.js"></script> <link rel="stylesheet" href="style.css"> </head> <body> <section class="sect--1"><div></div></section> <section class="sect--2"><div></div></section> <section class="sect--3"><div></div></section> <section class="sect--4"><div></div></section> <section class="sect--5"><div></div></section> </body> </html>
And I wanted a function with the object as parameter, which is the element the mouse is over, to be called every time the mouse goes over one of the <section> elements. Here's how I tried to achieve it by javascript:
window.onload = function() {
/* Global Vars Declaration */
sects = sectDefine();
/* Events Declarations */
for (section in sects){
section.onmouseover = debug();
}
}
/* That function will put each <section> element as an object inside an array that will be returned to whatever called it */
function sectDefine(){
var nextSect = true;
var num = 1;
var sectClass;
var section;
var sects = [];
while (nextSect) {
var sectClass = 'sect--' + String(num);
var section = document.getElementsByClassName(sectClass);
if (section[0] != undefined){
sects.push(section[0]);
num = num + 1;
} else {
var nextSect = false;
}
}
return(sects)
}
/* That function is obviously not asking for any parameter right now, but it's being called for each event that is declared (for each section.onmouseover = debug() on the event window.onload) and never once the mouse is actually over one of the <section> elements that was supposed to call this function .onmouseover */
function debug(){
console.log('sup');
}
If I declared for each <section> element a function to be called .onmouseover, like
sects[0].onmouseover = function (){ console.log('sup') };
sects[1].onmouseover = function(){ console.log('Thats 1') };
/* And there it goes for each item inside the sects array */
It would have worked properly (because I tried and it did work properly; it called the function it's assigned to every time the mouse was over the specified object, and not when the page loaded), but it's not working with the actual code, it is, instead, calling the function instantly after the page is loaded and not when the mouse is actually over the object itself.
I omitted irrelevant lines of code and comment from the code that is right here, in this question; lines that worked independently and did not affect the DOM, variables or actual functionality in any way as much as I know, however the code I'm using will be available at this codepen sandbox, with no modifications AT ALL.
http://codepen.io/bakedCookie/pen/XbeYvO
I'm thankful for any constructive feedback provided.