Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial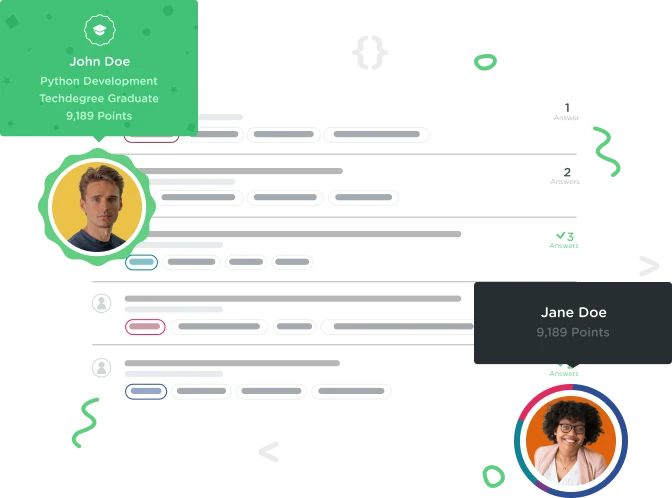

Julie Ogden
3,330 PointsWhy does "object[properties]" contain the values?
This just isn't very intuitive to me. I was wondering if anyone knew the thought process behind calling the object, and then using the properties variable to obtain the values in the object. Why not assign the values their own variable?
4 Answers

Gunhoo Yoon
5,027 PointsSo are you asking why should you access to values through an object while you can just access them through variables?
Like this?
//Based on video you watched plus think this as separate cases on separate file.
//Example1 your preferred way?
var name = 'Dave',
grades = [80, 85, 90, 95];
//Example2 what video tells you to do
var student = {
name: 'Dave',
grades: [80, 85, 90, 95]
};
//Example3 alternative
var student = ['Dave', [80, 85, 90, 95]];
If yes continue...
The difference between first and second is how data is maintained in future.
If you had 10 students, first approach requires 20 variables you should keep track of while second approach only need 10.
After a semester student's have updated grades. If you use first approach, you need to change all of them manually while second approach only needs a set of data with updated grades and function that will automate the update. I'm not saying it's not possible with first approach but it's significantly harder. (You will know why once you start learning method and constructor)
So you will work more and work harder if you didn't use object just because you prefer using first approach.
The difference between second and third is readability at this stage. (Again once you start learning more about object things will make sense)
If you want to access student's name, you can do student.name or student[name] for second approach while third approach needs to do something like student[0].
Imagine If your student has information like email, phone_number, address, and etc.... With second approach it is done by student.address, student[address] while third approach needs to do something like student[4]. Which one would make more sense when you suddenly encounter them in your code?
These are few reasons I can think of assuming you don't know the constructor patterns and methods.
So to wrap up your question, "Why do I need to use object[property] over plain variable" is because that is how you can access object's variable and using object is significantly better in many situation.
I might be completely misunderstanding your question, so in that case please let me know.

Jess Hines
5,411 PointsTo expand and reiterate, the variable in the for...in can be named everything, and by convention this is singular (e.g. "prop," "key" etc). So then, regardless of whether you're in a loop or not, you can always get the value for a specific key with object[key], and this will return the value for that key.
You cannot use dot notation in this case because what comes after the dot must be the actual name of the property, and 'key' (or 'prop' or 'value') isn't likely an actual key name on your object.
So, given the following object:
var myObject = {
color: 'blue',
shape: 'square'
};
Then, if you want to find out the color, you could use either myObject.color
or myObject['color']
but not myObject[color]
because whatever is in those brackets should be able to be evaluated as a string, and in this case it's a variable that you haven't defined.
However, if you wanted you could have another variable, say var myProp = "color"
then you could use myObject[myProp]
which is really all that's happening in the for...in loop; a variable is standing in for the property name (which is a string) so that the value that property points can be retrieved.
Hope that helps.

Julie Ogden
3,330 PointsThank you for your response! I was pretty deep into my thought-process when I wrote this question, so sorry if it doesn't make sense!
My question came about while learning about for ... in loops. Here is a very basic for ... in loop for example:
for (var properties in object) { console.log(object[properties]); };
The above loop logs the VALUES to the console. In my mind, I would think you would write console.log(object[values]) ;, or some such term that is directed at the values directly. This may not have an easy answer, I'm just curious if anyone knows why it is phrased this way.
Thank you again!

Gunhoo Yoon
5,027 PointsOh yeah sorry I completely misunderstood what you meant there.
See if this can help you.
First
If you are having problem with the loop variable name properties
you can simply change to your preferable such as
for (var value in object) {}
However, in this case the instructor used name called properties (it should actually be a property not properties to make more sense) instead of value because for ... in ...
loop actually picks up the property of an object and this is by JavaScript design.
Second
Then you can think "Why the hell a loop variable can't point to actual value?". That may make you type a lot less because you can directly refer to value instead of object[property]. However, you need to think about other cases where objects are usually accessed. For example,
Let's say you have two student objects with name and grades. If the only option to retrieve value is by calling object[value] you have to know exact value on every instance of student. But if you can access value through object's property which is common as long as that object is built from same template, the object[property] makes more sense because you are using common denominator rather than ever changing values.
Did this help?

Andrew Day
2,749 Pointsif you're wondering which to use after all of these explanations, i'd suggest the dot method.
Clovis Shropshire
7,139 PointsClovis Shropshire
7,139 PointsThis is a great answer! Very helpful amount of detail!