Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial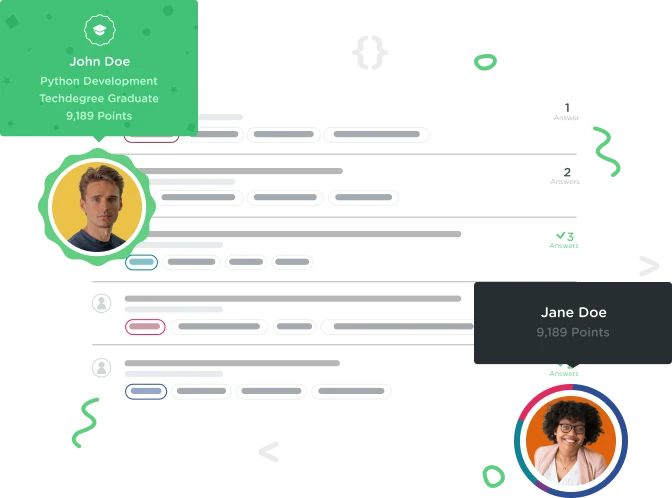
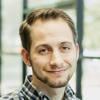
Wesley Goldsborough
4,824 PointsWhy does person[prop] access the key? It appears like that would access the property.
I read through the questions, and Julie Ogden's question is similar to mine, but I don't think her intended question was ever really answered.
I understand how the 'for in' loop works and functions, it just seems that the way the value of a property is accessed doesn't make sense. The below code will log each property's key belonging to an object named 'person'.
for (prop in person) {
console.log(person[prop]);
}
but since the variable 'prop' refers to the property (not the value) being iterated, I don't understand why person[prop] would not just access the property. I understand that person[prop] accesses the key, it just seems to me like it would access the property instead.
To wrap it up, it seems to me like both of the loops below would log the properties of an object named 'person', but in fact the first loop logs the properties, and the second loop logs the values.
for (prop in person) {
console.log(prop);
}
for (prop in person) {
console.log(person[prop]);
}
2 Answers
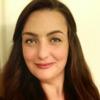
Jennifer Nordell
Treehouse TeacherHi there! When I was trying to wrap my head around this the first time, it was explained to me that you can sort of think of this like an array. I'm fairly sure you're familiar with arrays at this point. An array is an object in JavaScript with predefined keys going from 0 and counting upwards. So to get the value in an array you need to point at that spot in the array by telling it the key.
This is the same concept with your Person object. But the big difference here is that you determine what the keys are named. I made a simple JavaScript snippet that you can run in the console to see how it works in an array.
var myArr = ["Hi", "there", "Wesley"];
for (key in myArr) {
console.log(key);
}
for (key in myArr) {
console.log(myArr[key]);
}
You'll see that the first for loop logs the numbers 0 through 2 because those are what the keys are actually named. And the second logs the values at those keys. Hope this helps!

Erik Nuber
20,629 PointsOne of the big things that he tries to push in the video is that the word prop could be anything. So keep that in mind
for (var iCanBeAnything in person) {
console.log(iCanBeAnything)
}
this would log out just the keys and it doesn't matter what the variable is.
for (var iCanBeAnything in person) {
console.log(person[iCanBeAnything])
}
this is saying that now you need the value for each key within person.
Wesley Goldsborough
4,824 PointsWesley Goldsborough
4,824 PointsThanks Jennifer that helped me understand better by making the comparison to an array. It also helped me start to understand how "everything in javascript is an object". I've heard people say that, but didn't really understand until you explained how an array is an object where the index values are the keys and the values are what you see visibly in the array code itself.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherWesley Goldsborough you're quite welcome! I wish I could take full credit, and moreover, I wish I remember who I heard that comparison from or where I read it. What I do remember was having one of those light bulb
moments 
Hanzo Akimaru
161 PointsHanzo Akimaru
161 PointsNice explanation :)