Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial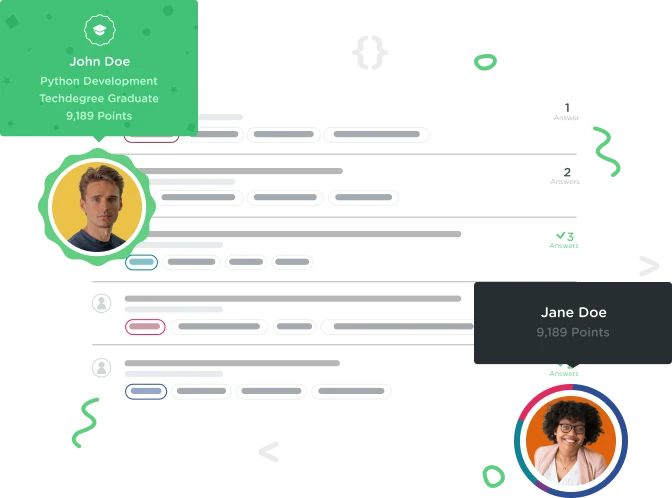

JASON LEE
17,352 PointsWhy does spaces array need to be multi-dimensional? Can't it be a single dimensional array?
The 'spaces' array is a multi-dimension array of rows and columns. And the array holds 'Space' objects. 'Space' object already contains a property with the x and y values, so it seems redundant that the 'spaces' array itself is also multi-dimensional, since we already should have the x and y values.
Am I missing something here?
2 Answers
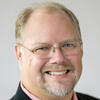
Jason Larson
8,361 PointsIn theory, you are correct. The problem is that while the space objects contain an x and y value, you have to populate those values with 2 loops, and since you're already going through the 2 loops, you might as well go ahead and create the spaces array as a virtual representation of the physical thing it represents. Yes, you could just add them in as one big long array, but it doesn't buy you anything, and it makes things more difficult later when you are updating the individual spaces. As with nearly all programming tasks, there is more than one way to accomplish the goal. The decisions are up to you as the developer about how it should be implemented. I actually would encourage you to try it your way (after you've done it the way the course has you do it and ensure that it's working), and see which way makes more sense to you, or which way is more efficient. Sometimes things are done a certain way just because it makes more sense to the developer's way of thinking about things, or because they don't know a different way. Testing different methodologies is a great way to cement the understanding in your learning.

Daoud Merchant
5,135 PointsAs additional information to the above excellent answer, and in case it might benefit anyone else, it may help to know that accessing an index in an array is an extremely quick operation, so by creating a 2d array one can use x
and y
coordinates to access a given value near instantly.
Imagine a single-dimensional array of spaces with the x
column coordinate, y
row coordinate and, say, a unique name
(just to give each item something unique to access), generated with nested for
loops as per the Treehouse exercise. Below is what that would look like for a 2x2 grid:
const spaceArray = [
{ x: 0, y: 0, name: "Sam" },
{ x: 0, y: 1, name: "Gail" },
{ x: 1, y: 0, name: "Ben" },
{ x: 1, y: 1, name: "Ellie" }
];
How would you access the name of the object with an x
of 1 and y
of 0? As Mr. Larson states, such experimentation is well worth the time.
Now as a 2d array as per Ms. Boucher's solution:
const spaceArray = [
[
{ x: 0, y: 0, name: "Sam" },
{ x: 0, y: 1, name: "Gail" },
],
[
{ x: 1, y: 0, name: "Ben" },
{ x: 1, y: 1, name: "Ellie" }
]
];
As the x
will always equal the index of the nested array and y
will always equal the index of the object within the nested array, now it's as simple as:
spaceArray[1][0].name; // "Ben"
Hope this helps!