Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial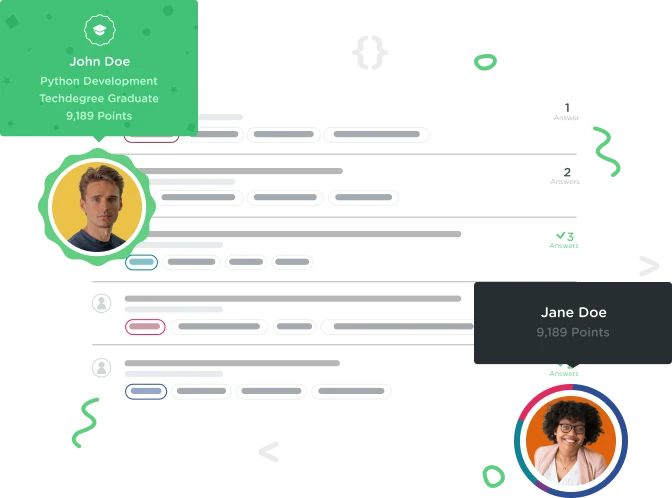

ramseydennis
4,530 PointsWhy does the browser know to "guess" the number based on this code?
Hey everyone,
My apologies if this seems obvious but I don't understand exactly how the browser knows it should guess a number here - what part of the code is commanding it to guess?
I don't understand how "guess=getRandomNumber(upper)" is telling it to guess, when it seems that getRandomNumber(upper) is what is a function that is in fact generating the random number that the browser is going to eventually be instructed to guess.
I must be thrown off of course, since the code obviously works.
Thanks in advance.
var upper = 10000;
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while (guess !== randomNumber) {
guess = getRandomNumber(upper);
attempts +=1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p> It took the computer " + attempts + " attempts to get it right.</p>");
6 Answers

Erik Nuber
20,629 PointsInitially "guess" is set to nothing so it is a variable that does not equal the randomNumber within the while loop.
Because of this, the loop is allowed to execute at least a single time.
This line of code
guess = getRandomNumber(upper);
now assigns the variable "guess" an actual number between 1 and 10,000 or whatever you set the upper variable to.
Once the variable "guess" is assigned a number, the While loop looks again. Guess is now assigned a random number because the function for the random number was called and, it is comparing it to a fixed variable random number that was assigned outside of the loop. If they do not match, it happens again and again. If it does match, that's it.
If it helps to think about it this way, reduce the number of assigned variables and replace them with numbers
function getRandomNumber(x) {
return Math.floor( Math.random() * 10000 ) + 1; /*this returns a random number when called*/
}
var x; /*used just to call the function can be anything*/
var guess; /*unassigned variable right now*/
var attempts = 0;
while ( guess !== 5345 ) { /*always executes at least once*/
guess = getRandomNumber( x ); /*guess is assigned a random number */
attempts += 1
}
document.write ("<p>The number we are trying to find is " + 5345 + ".</p>");
document.write (" <p>It took the computer " + attempts + " times to hit that number.</p>");
now we no longer have a randomNumber to compare to, instead we are giving it a specific number to compare to. The function is called with "x" variable which can be anything you want as the only thing that matters is that the function is called correctly and again, sends back a number.
I don't know if decreasing the variables and simplifying the code will help you at all. Because I think the people that have answered have done a good job and perhaps it is more a misunderstanding what you are looking to have explained.

Markus Ylisiurunen
15,034 PointsHi Josh!
I'll try to explain it again better. I assume you understand basic JavaScript variables, loops and functions. So first let me write a simpler version of the previous code.
/* Number that the computer tries to guess */
var numberToGuess = randomNumber();
/* Current guess */
var currentGuess = null;
/* Function that generates a random number between 1 and 10 */
function randomNumber() {
return Math.floor(Math.random() * 10 ) + 1;
}
/**
* Loop which acts as an instruction for the computer to
* guess until it has guessed the right number
*/
while (currentGuess !== numberToGuess) {
currentGuess = randomNumber();
}
/* Horray! Computer has guessed the number when it gets here. */
So the numberToGuess
variable will be generated when the code first starts to execute. This is the number that the computer will try to guess.
currentGuess
is the variable that holds computer's current guess. Here's how the program will execute step by step.
Step 1. Initialize variables
Step 1-1. numberToGuess
will become an int between 1 and 10
Step 1-2. currentGuess
will initially be null
Step 2. Start while loop (loop until currentGuess is the same as numberToGuess)
Step 2-1. Check if currentGuess is not the same as numberToGuess
Step 2-2. If not, run while loop
Step 2-3. Guess a new number and set it to currentGuess
I hope you can know see what tells the computer to "guess" a new number over and over again. It's the while loop that does it. It'll only get out from the while loop after it has guessed the right number.
Hopefully this explains it better than my previous explanation!

Markus Ylisiurunen
15,034 PointsHello ramseydennis!
I'll try my best to explain it to you. So looking at the variables you can see that it generates a randomNumber when the software initially starts executing. Now randomNumber is some number the computer tries to guess.
So next we have the while loop which will loop until the condition is false (when randomNumber is the same as the guess). So initially they won't be and we'll get into the loop. There the computer generates a new random number and assigns it to guess. Then the loop will check again if the randomNumber is the same as the guess. If it isn't, the computer will keep guessing until it finds the right number. When it does, the loop will stop and you'll show the results.
Hopefully it helps!

Josh Curtis
7,782 PointsI have the same question. I think we understand how a random number is generated but what tells the computer to "guess"? And how does it know what we're trying to guess? From my very uneducated point of view it seems like there should be some sort of instructions assigned to the variable guess. Is guess a keyword that always tells the computer to generate a number?

Josh Curtis
7,782 PointsThank's for trying to explain! I completely understand the process of the code and how it generates a random number and so on. Going back to the original code:
function getRandomNumber(upper) { return Math.floor( Math.random() * upper ) + 1; } This makes total sense. The variable getRandomNumber is given a value by the Math.random code.
What I'm not grasping is why we can just throw a variable into the code and the computer automatically knows that it is supposed to generate a number for it.
while (guess !== randomNumber) {
guess = getRandomNumber(upper); attempts +=1;
}
Guess doesn't have any instructions to generate a number. It's just there. It has instructions to compare the number it chooses to the random number. Maybe when a variable is compared to a number, the computer automatically knows to choose a number? This is incredibly hard to explain what I'm thinking and I'm sure there's a perfectly easy answer to it. Thanks for your time.

Josh Curtis
7,782 PointsOk I think the light bulb finally came on. I haven't had much practice with these so I was thinking about it the wrong way. So the function assigns a number to randomNumber. Then the while loop calls the random number function again and assigns that number to guess. This repeats until guess=randomNumber. Thanks for the help! Sorry to take over your question ramseydennis. Hopefully it helps you too.
Kevin Baxter
5,599 PointsKevin Baxter
5,599 PointsI sware a light bulb just went off...thank you so much!!!