Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial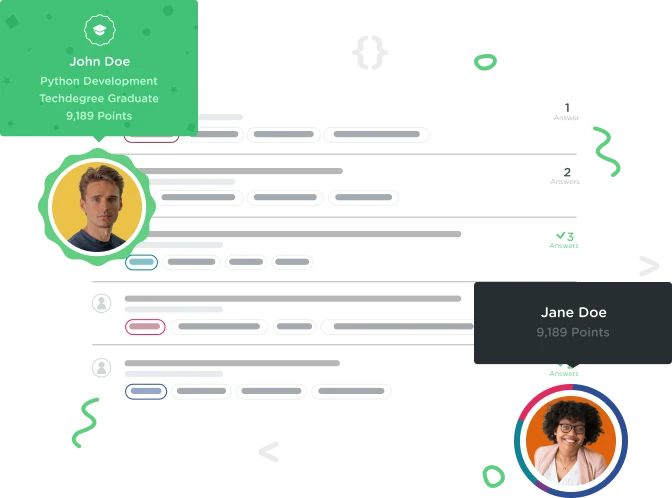

Sean Henderson
15,413 PointsWhy does the challenge throw an error if I don't user the strict not equals operator?
I have some code that satisfies the condition - user is prompted infinitely until 'sesame' is entered, at which point the page is displayed; however, the Challenge Editor is throwing:
Bummer! Use the strict not equals operator -- !== -- to compare the variable
secret
and the string 'sesame'.
I don't understand why I need to use the strict not equals operator :/ To paraphrase what I have in pseudo code:
authorized = false while authorized === false prompt user if user input === 'sesame' // STRICTLY_EQUALS authorized = true show document and write to it
var authorized = false;
while (authorized === false) {
var secret = prompt("What is the secret password?");
if (secret === "sesame") {
authorized = true;
}
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>

Sean Henderson
15,413 PointsThanks @andrewrobinson3, but I'm not using a non-strict operator to check if (secret === "sesame")
, I'm using the strict equals (===
). What gives?
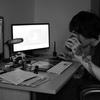
Andrew Robinson
16,372 PointsAh jeez I've just woken up sorry, I think the problem is the challenge is asking for a while loop only, so instead of making a flag and changing that with an if statement you can just say
while (secret !== 'sesame') {
secret = prompt("What is the secret password?");
}
Way less code for the same task.

Sean Henderson
15,413 Points@andrewrobinson3 I just figured this out some refactoring. Was throwing too many SLOC at the problem...
Thanks for the quick response, have a great day!
1 Answer

Sean Henderson
15,413 PointsThe Challenge Editor was expecting a much more elegant solution - putting the comparison of secret
and 'sesame' directly into the conditional of the while
loop.
while (secret !== 'sesame') {
secret = prompt("What is the secret password?");
}
This is why it was expecting the strict not equals operator -- !==
--. I overcomplicated things by nesting an if
block in the loop :)
Andrew Robinson
16,372 PointsAndrew Robinson
16,372 PointsThe challenge is just trying to enforce best practices, a non-strict operator is something to use when you need the side effect of using it I think.