Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial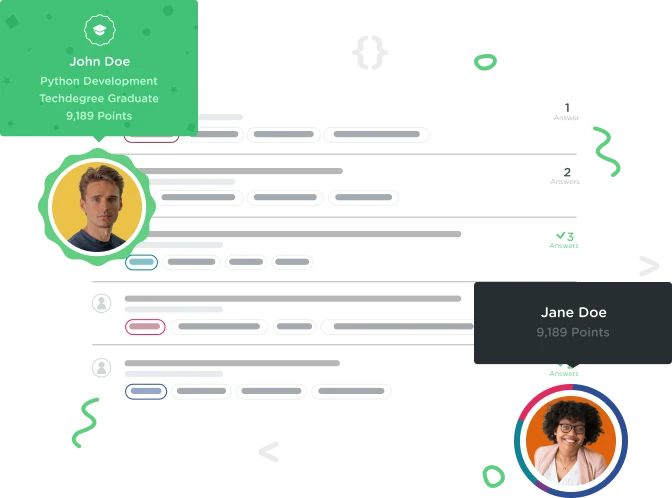
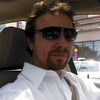
Ron L
6,463 PointsWhy does the if statement 'if divisible_by_3?(number)' come after calling the methods?
Here is the code: name = get_name() greet(name) number = get_number()
if divisible_by_3?(number) puts "Your number #{number} is divisible by 3" else puts "Sorry, your number #{number} is not divisible by 3." end
We're using the if statement on divisible_by_3? method with the number parameter, which was returned in the defined method above it, so I don't see why we can't place the if divisible_by_3? statement before calling the methods, like all the other parts of the code.
And, what is the intuitive part that would tell us that this portion needs to come after the calling of the methods?
2 Answers
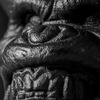
Michael Prince
23,132 PointsRon,
It looks like this has to come after the methods because the get_number() method is converting the answer to an integer (to_i). Once the answer is an integer you can then perform the divisible_by_3? method.

Jeramae Bohol
4,550 PointsRon,
I hope this other version helps you. It works the same way as the video but easier to understand.
def get_name()
print "Enter your name: "
return gets.chomp
end
def greet(name)
puts "Hi #{name}!"
if (name == "Jason")
puts "That's a great name!"
end
end
def get_number()
print "What number would you like to test? "
return gets.chomp.to_i
end
def divisible_by_3?(number)
if (number % 3 == 0)
puts "You number is divisible by 3!"
else
puts "Your number is not cleanly divisible by 3!"
end
end
name = get_name()
greet(name)
number = get_number()
divisible_by_3?(number)