Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial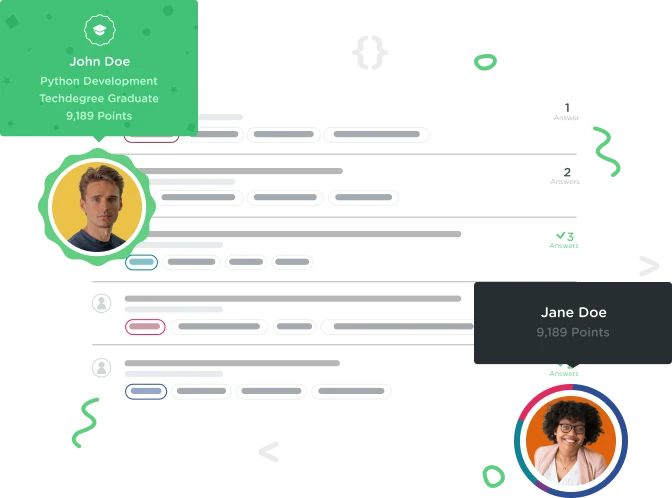
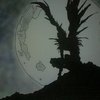
Lucas Santos
19,315 PointsWhy does the jQuery events such as focus and keyup not take methods with () at the end and just the method name?
The code:
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
var $username = $("#username");
//Hide hints
$("form span").hide();
function isUsernamePresent() {
return $username.val().length >0;
}
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching() && isUsernamePresent();
}
function passwordEvent(){
//Find out if password is valid
if(isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if(arePasswordsMatching()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$("#submit").prop("disabled", !canSubmit());
}
function usernameEvent() {
if(isUsernamePresent()) {
$username.next().hide();
} else {
$username.next().show();
}
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
$username.focus(usernameEvent).keyup(usernameEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
A question out of curiosity here, why is it at the bottom with the focus and key up events where it takes the methods does the method name not have the function parenthesis after them ()
Meaning why is it like this:
$password.focus(passwordEvent)
and not like this:
$password.focus( passwordEvent() )
I thought whenever you call a function / method anywhere you need the parenthesis after them ()
Whats the acception here?
2 Answers
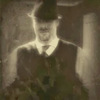
Joel Smith
14,779 PointsIn this particular case, you're referring to the functions from within the methods keyup and focus, not calling them. When referring to a function, calling it with the parenthesis is unnecessary.
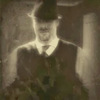
Joel Smith
14,779 PointsI think that this case is particular. The aforementioned functions are being used as arguments within methods here. In this case, the parenthesis are not necessary. Just a "function" of JS (pun intended).
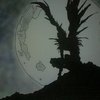
Lucas Santos
19,315 PointsSo let me ask you this. If I wanted to could I use the parenthesis () in focus like this:
$password.focus( passwordEvent() );
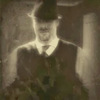
Joel Smith
14,779 PointsTo be honest, I'm not sure, because I've never tried it. Try it out.. or check the documentation, perhaps there's more info on syntax for using a function as an argument for a method.
Lucas Santos
19,315 PointsLucas Santos
19,315 Pointshmm I kind of dont understand because if you look at the code example there are some functions that aren't being called and use the () parenthesis.
For instance here in both of these examples they are not calling the function but referring to them:
Here it's referring the functions as a second parameter in prop()
As you can see in those examples they are not calling the functions but referring to them. So does this mean that only applies inside a method?