Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial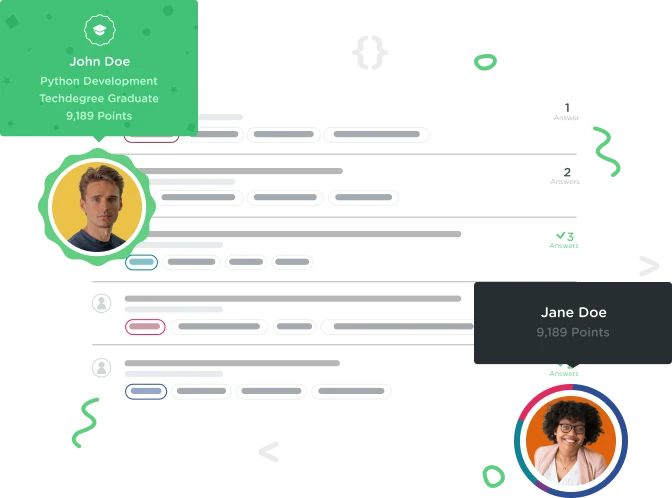

Randy Cole
Courses Plus Student 2,494 PointsWhy does the "load" method return nothing?
I understood that void is used when nothing is returned but aren't we returning an integer when declaring mPezCount = MAX_PEZ which is set to 12
1 Answer
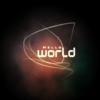
Kristian Terziev
28,449 PointsNot exactly. Yes, void is used when nothing is returned and therefore the method load doesn't return anything. It just does something. I'll give you some examples so you can understand. Let's say you have the following class:
public class Dog{
private String name;
public void bark(int times) {
//the dog barks "times" times
}
public int getHumanAge(int dogAge) {
//For the first two years, a dog year is equal to 10.5 human years. Meaning in 2 years the dog will be 2 dog years and 21 human
return dogAge*10.5; // let's assume it's not just for the first two years
//you get the age of the dog
}
}
And then you create an instance of that class:
Dog husky = new Dog();
Now what can the husky do? Simply - bark. Yes it has a name(not relevant) and age (notice it's not a member variable) which you can access through the method, but the husky itself can't speak to you and tell you its age, can it? Therefore it's only ability is to bark. When it barks you don't get the word back written on a paper, do you? No, you just hear the sound. That's why bark is of type void - because it does something and returns nothing.
Now what about getHumanAge()? It does something too, right - calculates the dog's age in human years. Why is it not void? Because after the calculation, it gives you back that integer to work with. Therefore you can do something like this:
int humanAge = husky.getHumanAge(2);
System.out.printf("My husky is %s human years old", humanAge); // 21
But you CANNOT do this:
<type> bark = husky.bark(3);
System.out.printf("My husky barked %s times", bark);
It doesn't make any sense. You can't assign the method to anything because it return nothing.
So coming back to your code. The method load simply refills the pez dispenser and it's done. It doesn't return you the number of candies inside the dispenser, it return the moment it did it. It just simply obeys your order without any question - Therefore void.
I just made up that example with the dog, so if it's confusing please let me know. I hope that clears it up.
Randy Cole
Courses Plus Student 2,494 PointsRandy Cole
Courses Plus Student 2,494 PointsOk that makes sense... I believe what's being explained is, and please correct me if I'm misinterpreting a bit, whenever a method absolutely does one thing without any flexibility in what it can return it's basically returning nothing and only carrying out a sort of direct command as opposed to a command for an answer?
Kristian Terziev
28,449 PointsKristian Terziev
28,449 PointsWell I'm not sure I absolutely understood you (english is not my native language), but void is not limited to doing only one thing. It can do as much things, as you want. You can even call another method inside it. It just comes, does it's thing and goes away. Yes it executes a command and that's it.
One more note - Methods that return something (doesn't matter what) also can do more than one thing.
Randy Cole
Courses Plus Student 2,494 PointsRandy Cole
Courses Plus Student 2,494 PointsThat makes sense, Thanks I really appreciate the clarity!