Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial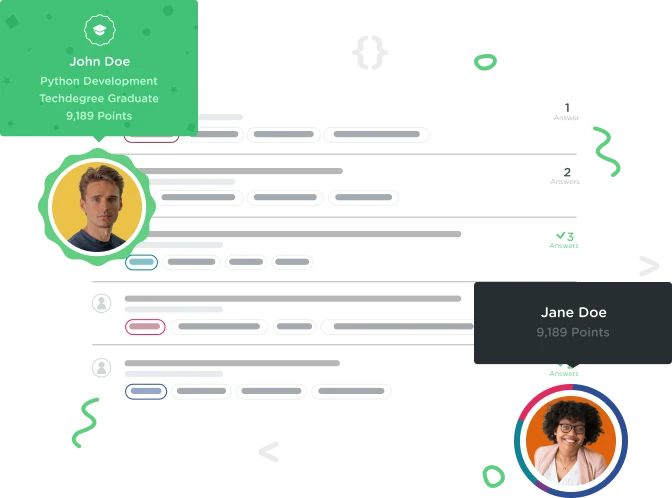
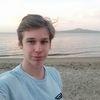
Máté Tóth-Csák
7,509 PointsWhy does the name of the property not show up in my code? (Using for in loop)
Hello Folks.
Newbie here. First time learning about JS.
I had my own solution for this challenge which looked like this:
var students = [
{
name: "George",
track: "Android",
achievement: 4,
points: 3054
},
{
name: "Sarah",
track: "IOS",
achievement: 3,
points: 2143
},
{
name: "Michael",
track: "Web",
achievement: 9,
points: 8954
},
{
name: "Clarke",
track: "Front End Development",
achievement: 5,
points: 6431
},
{
name: "George",
track: "Back End Development",
achievement: 9,
points: 5310
}
];
for (key in students) {
document.write(key, ": ",students[key].name, "<br>");
document.write(key, ": ",students[key].track, "<br>");
document.write(key, ": ",students[key].achievement, "<br>");
document.write(key, ": ",students[key].points, "<br><br>");
}
I thought if I use the key word the page will print out the name of the property as well.
But instead of "Name: Sarah" I got "0: Sarah" printed out.
Can somebody please explain to me how this works exactly.
I understand, that "key" would not be a good solution, since it is repeated in every line, so it should give out the same value. (like: "name 4x under each other, and not name, track achievement, points)
I passed the challenge, with replacing key with simple strings, but you definitely can't do that if there are over 100s of properties.
Should we combine a simple for loop with the for in loop?
3 Answers

andren
28,558 PointsIf you were looping over a student object then key
would indeed reference the property name, but you are not looping over a student object, but instead looping over the students array.
When you loop over an array you pull out the indexes of the items in the array, not the properties of the items inside the array. So when you use students[key].name
you are essentially just pulling out one object from the array based on the current index and then pulling out the value of the name property.
You can get the behavior you desired pretty simply by nesting a loop which loops over the individual student objects within the existing array loop like this:
for (key in students) {
var student = students[key] // Assign the current student to a variable called student
for (property in student) { // Loop over the properties of that student
document.write(property, ": ",student[property], "<br>");
}
}
You can of course do the above without creating the student
variable, I just created that to make the code a bit easier to read.
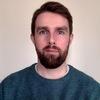
Richard Lambert
Courses Plus Student 7,180 PointsHello Mate,
An integer value is being returned because you're iterating over an array of objects. Placing these student objects within a students
object and associating each with a unique key such as a username (potential for duplication with actual names e.g. George in the code) would result in key
returning a value more in line with what you expected:
var students = {
'username1': {
name: "George",
track: "Android",
achievement: 4,
points: 3054
},
'username2': {
name: "Sarah",
track: "IOS",
achievement: 3,
points: 2143
},
'username3': {
name: "Michael",
track: "Web",
achievement: 9,
points: 8954
},
'username4': {
name: "Clarke",
track: "Front End Development",
achievement: 5,
points: 6431
},
'username5': {
name: "George",
track: "Back End Development",
achievement: 9,
points: 5310
}
};
for (key in students) {
document.write(key, ": ",students[key].name, "<br>");
document.write(key, ": ",students[key].track, "<br>");
document.write(key, ": ",students[key].achievement, "<br>");
document.write(key, ": ",students[key].points, "<br><br>");
}
Hope this helps
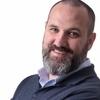
julien moulis
7,580 PointsHi, I think that for in works on object. Here is a code with a for loop
for (var i = 0; i<students.length; i++){ console.log(students[i].name) }
You just need to add the rest
Hope it helps