Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial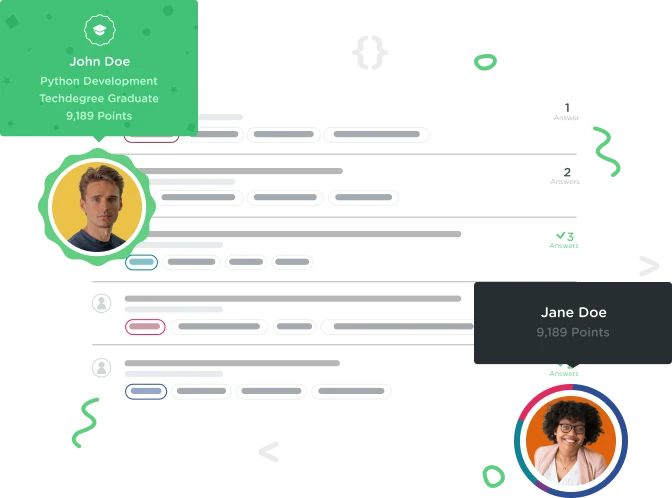
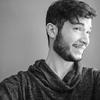
Damian Adams
21,351 PointsWhy does the order of the ''FizzBuzz'' and the ''Fizz'' statements matter in the code?
Why must the ''FizzBuzz'' ampersand ''if'' statement go before the ''Fizz'' ''else if'' statement in the code? I noticed that if the ''if...Fizz'' statement comes before the ''else if...FizzBuzz'' statement in the code the line ''FizzBuzz'' will not appear, even though one might think it should.
What does this reveal about the language? One would think that Swift could modify the ''Fizz'' entries into ''FizzBuzz'' but it apparently looks like it can't if phrased in this sequence. Only when ''if... FizzBuzz'' comes phrased first in the sequence does it appear in the compiled output.
Why is this so?
2 Answers
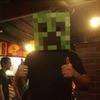
2btrzt9ior
15,232 PointsHi Damian,
If I understand your question correctly, you're asking about the Swift implementation of FizzBuzz, as shown in the following script:
for i in 1...20 {
if (i % 3 == 0) && (i % 5 == 0) {
println("FizzBuzz")
} else if (i % 3 == 0) {
println("Fizz")
} else if (i % 5 == 0) {
println("Buzz")
} else {
println(i)
}
}
You seem to be wondering why, if lines 2&3 and lines 4&5 switched positions, the console wouldn't print "Fizz" and then print "FizzBuzz" right after if both conditions were satisfied.
If this is what you were asking about, the answer is because the program will break out of the current if-else chain once a conditional statement has been satisfied. So if the conditions for the program to print "Fizz" were satisfied, then the program doesn't bother reading the else if statements below; it just skips down to the next if statement or, if there are no more, starts the next iteration of the for loop.

McKenna Rowe
2,930 PointsI had this same issue, i had the condition for 3 first, 5 second, then 3 and 5 last. I followed the logic of the exercise instructions which went in that order. But i moved the "divisible by 3 and 5" to the top and it worked. Xcode never gave me any errors either, which made it trickier. I'm not 100% sure how to always know the proper order. Does the condition with most complex requirements always go at the top?
Damian Adams
21,351 PointsDamian Adams
21,351 PointsAh, I see. I understand now. I imagine the language is configured this way to be more efficient on resources when running huge loops?
Great answer, thanks!
2btrzt9ior
15,232 Points2btrzt9ior
15,232 PointsI imagine the language is configured this way to be more efficient on resources when running huge loops?
Yes, efficiency is important, but even more than that, it works this way because you are designating mutually exclusive, branching paths for the program to take.
Maybe for the FizzBuzz script it would make sense to print both "Fizz" and "FizzBuzz", but imagine if your if statement was, for example, controlling the background color of an app or web page. Suppose that instead of printing "FizzBuzz" the background would be set to a purple color, instead of "Fizz" the background would be set to a blue color, and instead of "Buzz" the background would be set to a red color. This would be a terrible way to set background color, but bear with me for a moment.
What should happen? If the if statement worked the way you thought it did, then the script would try to set the background color to both purple AND red. This ruins the entire point of using if statements to make decisions.
If you did want the script to print both "FizzBuzz" and "Fizz", then you would use another if statement instead of an if-else statement, like so:
Now the program should look at each statement in turn and you should see it print both "FizzBuzz" and "Fizz" on the proper occasions.
Also, looking back at my explanation, I don't think I explained it properly. The program wouldn't break out of the for loop, it would break out of the current if-else chain. I apologize for misconstruing that and I'll edit my answer.