Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial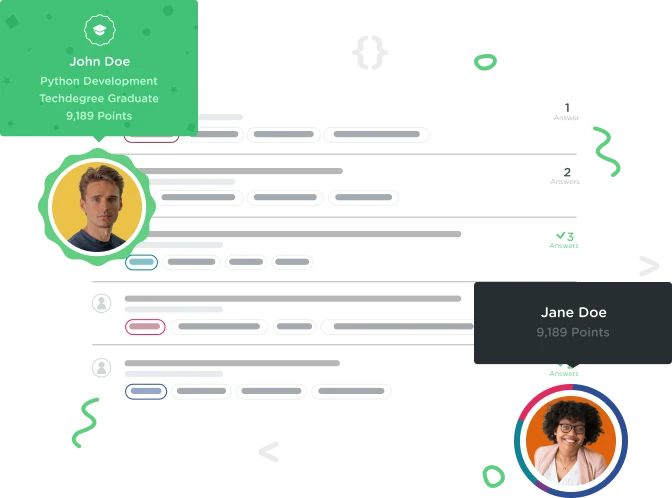
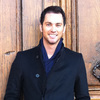
M Fen
9,858 PointsWhy does the = sign need to be on the += incriminator?
If you take the = sign of the += it runs forever. Why do you need the = sign? Just curious if anyone knows.
i = 0
string = "hello world"
while i <= 10
string = "hello world #{i}"
puts "i is now #{i}"
i += 1
end

Kyle Daugherty
16,441 PointsAndrew, you are spot on!
Cheers!
1 Answer
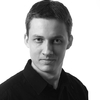
Maciej Czuchnowski
36,441 PointsThink of it this way:
Numbers, such as 1, 2 and 3, are constants that always have fixed values - 1, 2 and 3 accordingly (think "variables with fixed values and names that are exactly the same as values" - the constant named 2
has the value 2
). So if you do this:
1 + 2
You get the result 3, but you did not change the value of 1 or 2 at any point - if you check the value of 2 after this operation, it will still be 2. So similarly, if you create a variable x
and give it a value 5, and then do this:
x + 2
You will get a result of 7, but you did not modify the value of x. It is still 5, just like 2 remained 2 in the previous example.
The difference between constants and variables becomes noticeable when you try to modify the values. And to modify the values, you use the = symbol (it doesn't work the exact same way it does in simple math). This symbol modifies the value.
If you try to modify the value of x
, no problem. This will change the value of x
to anything you want:
irb :002 > x = 5
=> 5
irb :003 > x = 7
=> 7
But if you try to change the value of 2, it will not work and will give you an error.
irb :001 > 2 = 3
SyntaxError: (irb):1: syntax error, unexpected '=', expecting end-of-input
2 = 3
^
Because 2 has a constant value of 2 and cannot be changed.
This:
i += 1
Is a shorthand for this:
i = i + 1
And reads "take the current value of i, add 1 to it, and make the result the new value of i". The right-hand side is always evaluated first and the result is then assigned to the variable given on the left-hand side.
Andrew Gay
20,893 PointsAndrew Gay
20,893 PointsThe = character in most programming languages is known the assignment operator.
So i + 1 will output whatever i + 1 is (In this case you assigned i to 0, so 0 + 1 = 1) but not actually assign that output to any variable (such as i). Instead, the code would continue through the loop outputting 1 infinity but again not actually assigning that value to i and as i remains 0 and is always less than 10 the loop would continue.
Edit: Had to edit this a few time in attempt to make it more clear but hope this helped! Also thank Kyle Daugherty for the verification!