Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial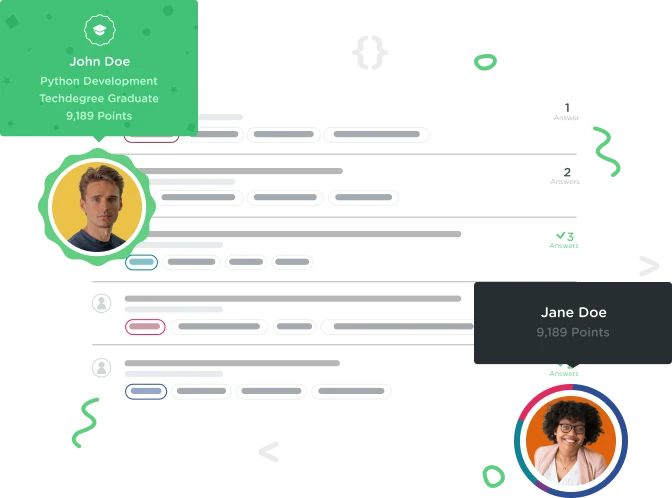

jakubhirner
14,784 PointsWhy does the size of JSON change?
import Foundation
let json = """
{
"name": "Pasan",
"id": 1,
"role": "Teacher"
}
""".data(using: .utf8)! // this is 59 bytes
struct Employee: Codable {
let name: String
let id: Int
let role: String
enum CodingsKeys: String, CodingKey {
case name = "name"
case id = "id"
case role = "role"
}
init(form decoder: Decoder) throws {
let container = try decoder.container(keyedBy: CodingKeys.self)
self.name = try container.decode(String.self, forKey: .name)
self.id = try container.decode(Int.self, forKey: .id)
self.role = try container.decode(String.self, forKey: .role)
}
}
let decoder = JSONDecoder()
let employee = try! decoder.decode(Employee.self, from: json)
let encoder = JSONEncoder()
let encodedEmployee = try! encoder.encode(employee) // this is 40 bytes
But when we decode it do employee and then back to JSON the size decreases to 40 but not back to 59 why is this?
1 Answer
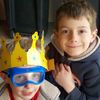
Chris Stromberg
Courses Plus Student 13,389 PointsYou have a lot of whitespace around your initial JSON object.
let json = """
{"name":"Pasan","id":1,"role":"Teacher"}
""".data(using: .utf8)! // this is 40 bytes
jakubhirner
14,784 Pointsjakubhirner
14,784 PointsThanks!