Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial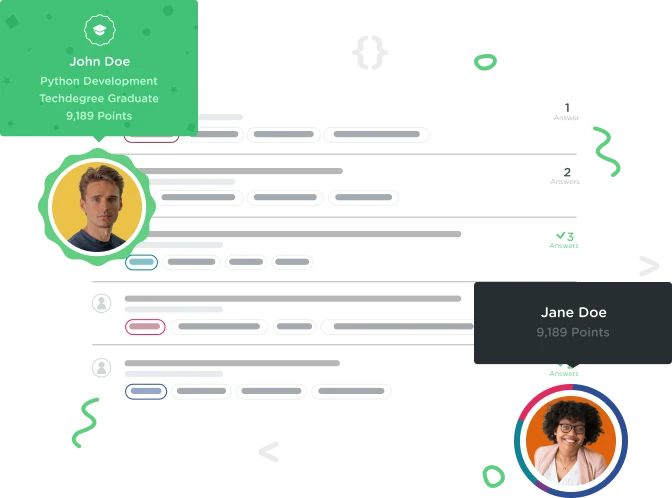

David Clausen
11,403 PointsWhy does the teacher use &block and yield?
During this monster class he uses &block and then uses the yield instead of block.call
# 1 This works cause yield is an implicit block call not using a Proc.new
def say
print "#{name} say..."
yield
end
# 2 this works cause yield is an implicit block call, but &block is actually never used
def say(&block)
print "#{name} say..."
yield
end
# 3 this works cause &block implicitly passes the block into a variable named block, and then #calls the block with block.call
def say(&block)
print "#{name} say..."
block.call
end
Why does the teacher use the example 2? &block call is far as i am aware is useless if not actually using the variable block he passed in. yield is implicit and already bring in the block that the method was called from.
Is there something I am missing or is the teacher just use to a habit that is just incorrect(thought not breaking anything)?
2 Answers
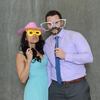
Christopher Aporta
24,258 PointsHey David Clausen,
As far as I understand it, the use of the explicit &block
pattern differs from the more implicit yield
in that the former creates a Proc (a Ruby object), while the latter does not (blocks are one of the few things in Ruby that are NOT objects). This has a couple implications.
One advantage of using &block
is that since it creates a Proc object, that object can then be stored in a variable (which you could then use .call
on). A disadvantage of this pattern though, is that it's a bit less efficient because it requires memory to store a newly created object. Implicitly using yield
does not create an object, and therefore does not require memory to do so.
So to your question about using them in tandem, it seems a bit redundant to me. Since we're negating the memory efficiency of yield
by using the &block
parameter to create a Proc, we should probably either use &block
with block.call
or yield
with mutual exclusivity. I could certainly be missing something, but I just don't see an advantage to the pattern used in this video.

David Clausen
11,403 PointsAfter researching it better i came up with using &block with yield makes reading the code easier as you dont have to look for yield to know immediately that method takes a block.
Does anyone have a different answer or can expand on it?
def say(&block)
print "#{name} say..."
yield
end