Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial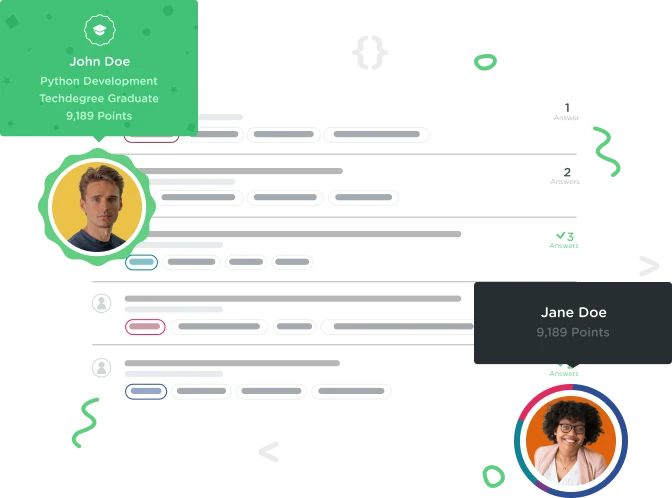

Amanda Kim
4,450 PointsWhy does this always print, "No Result Found"?
Even if I type existing student's name on the prompt, it always prints "No Result Found".
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jody',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student recprds: type a name [Jody](or type "quit" to end)');
if (search===null || search.toLowerCase()==='quit') {
break;
} else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message+=getStudentReport(student);
print(message);
} else if (student.name !== search) {
print('No Result Found');
}
}
}
}
4 Answers
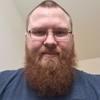
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsDo you have the array with student names in a different file?
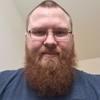
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsTo be honest I can't see the problem anywhere - the only thing might be if you are writing "jody" and the name in the array is "Jody" you can have a problem.
try add .toLowerCase or .toUpperCase to your !== search
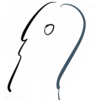
akak
29,445 PointsHey,
I've answered similar question here: https://teamtreehouse.com/community/code-only-works-when-the-last-students-name-is-entered-why
Your loop runs for every student so when it founds the right one prints it, but after that it still runs and prints no results found for every other student that passes else if condition (so basicly every other student apart from the one you are looking for).
Cheers!

Amanda Kim
4,450 PointsThank you. I read your answer to the post and helped me a lot. So, listening to your advise, I added break statement:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student recprds: type a name [Jody](or type "quit" to end)');
if (search===null || search.toLowerCase()==='quit') {
break;
} else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name.toLowerCase() === search.toLowerCase() ) {
message=getStudentReport(student);
print(message);
break;
} else if (search.toLowerCase() !==student.name.toLowerCase()) {
message='No Result Found';
print(message);
}
}
}
}
But it has other problem. When there are 2 students who have same name, (Jody for me,) it only prints the first Jody. How can I print both students' information at the same time?
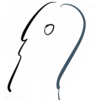
akak
29,445 PointsYou can try adding found users to an array, and after for loop ends print each of them. In that case you can get rid of the else statement and brake. You'll add only the "good" users to the array, and skip the wrong ones. After loop ends if the user or multiple users were found you will print them, else you will print no results found. I didn't check if the code works, but it should do the trick.
while (true) {
search = prompt('Search student recprds: type a name [Jody](or type "quit" to end)');
if (search===null || search.toLowerCase()==='quit') {
break;
} else {
var foundStudents = [];
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
foundStudents.push(student);
}
}
if (foundStudents.length > 0) {
foundStudents.forEach(function(student) {
print(getStudentReport(student));
});
} else {
print('No Result Found');
}
}
}

Amanda Kim
4,450 PointsThank you for your answer.
I managed to solve the problem following your advise:
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
var message = '';
search = prompt('Search student recprds: type a name [Jody](or type "quit" to end)');
var foundStudents=[];
if (search===null || search.toLowerCase()==='quit') {
break;
} else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name.toLowerCase() === search.toLowerCase() ) {
foundStudents.push(student);
}
} if (foundStudents.length>0) {
for (var j=0; j<foundStudents.length; j++) {
message+=getStudentReport(foundStudents[j]);
print(message);
}
} else {
print('No Results Found');
}
}
}
I appreciate your help!
Amanda Kim
4,450 PointsAmanda Kim
4,450 PointsYes, I do. I just added it. Sorry for not including it