Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial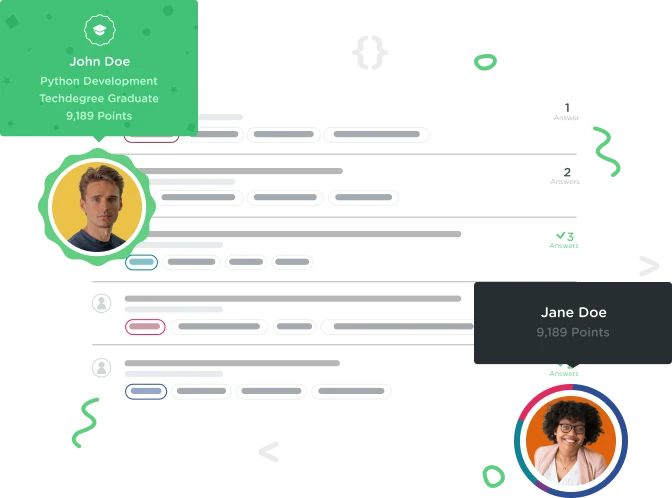
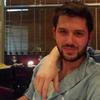
Nir Frank
3,578 PointsWhy does this code always return the same number?
Complete beginner here. I've got the following code:
var minNumInput = prompt("Enter a number");
var maxNumInput = prompt("Enter a 2nd number");
var minNum = parseInt(minNumInput);
var maxNum = parseInt(maxNumInput);
var randomNumber = Math.floor(Math.random() * (maxNum - minNum) + minNum);
for (var i = 0; i < 20; i++) {
if (i < 20) {
document.write(randomNumber + "<br>");
};
};
This just returns 20 instances of the same number. Going further, if I just copy and paste:
console.log(randomNumber);
a couple of times in my code, I'll still get a repeating sequence of the same number. HOWEVER, if I copy and paste something like this:
console.log(Math.floor(Math.random() * (10 - 1) + 1))
and repeatedly enter it inside my JavaScript console, each output IS random. What gives?
1 Answer
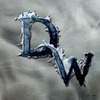
Hugo Paz
15,622 PointsHi Nir,
The reason you are getting the same number 20 times is because you only run the random function once and then print the result 20 times.
So what you want to do is to call the random function each iteration, like so:
var minNumInput = prompt("Enter a number");
var maxNumInput = prompt("Enter a 2nd number");
var minNum = parseInt(minNumInput);
var maxNum = parseInt(maxNumInput);
for (var i = 0; i < 20; i++) {
if (i < 20) {
var randomNumber = Math.floor(Math.random() * (maxNum - minNum) + minNum);
document.write(randomNumber + "<br>");
};
};
This will calculate a new value every iteration.
Nir Frank
3,578 PointsNir Frank
3,578 PointsHi Hugo Paz ! I was about to press "enter" on another comment - after staring at my screen I realized that was my problem. I came up with following solution, I would like it if you told me which is better:
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsI would do it differently. Something like this:
I prefer to separate the logic and the display.
Nir Frank
3,578 PointsNir Frank
3,578 PointsThis is awesome, as soon as I pressed enter on that I was trying to do just that - separating the document.write from the function into the for loop. I was missing the "return" (..key? property? function? object? whats the proper term?). I'm a little excited that my instincts turned out to be right. Also, good to know the proper terms for logic and display.. that, well, that makes a lot of sense :)
Thank you very much Hugo Paz for your quick replies!