Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial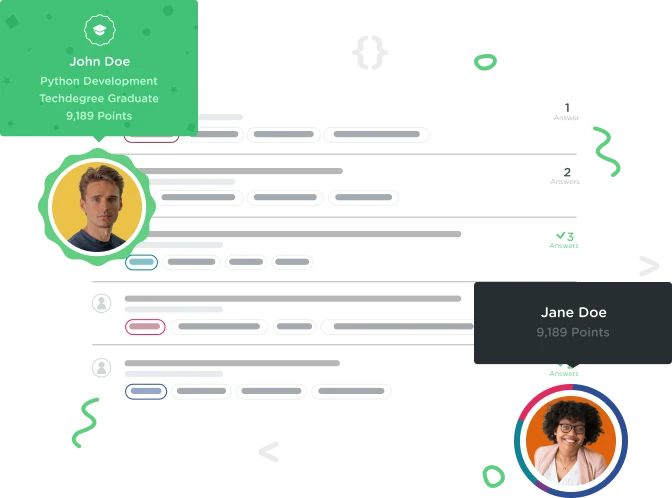

Benjamin Hobbs
15,195 PointsWhy does this code fail, and worse breaks task 2 of 3? I've tried it a few different ways and none of them works.
Have tried with and without the pk, blocks, reverse_lazy - also if I delete the entirety of my delete template the second task still fails, so maybe it's a bug?
from django.views.generic import ListView, DetailView, CreateView, UpdateView, DeleteView
from . import models
class ArticleList(ListView):
model = models.Article
class ArticleDetail(DetailView):
model = models.Article
crud_fields = ('title', 'body', 'author', 'published')
class ArticleCreate(CreateView):
fields = crud_fields
model = models.Article
class ArticleUpdate(UpdateView):
fields = crud_fields
model = models.Article
class ArticleDelete(DeleteView):
fields = crud_fields
model = models.Article
{% block body_content %}
<form method='POST'>
{% csrf_token %}
{{ form.as_p }}
<input type='submit' class="btn btn-primary" value='Save'>
</form>
{% endblock %}
{% block body_content %}
<h1>{{ article.title }}</h1>
<form method='POST'>
{% csrf_token %}
<input type="submit" class="btn btn-danger" value="Delete"> <a href="
{% url 'articles:detail' pk=article.pk %}">Cancel</a>
</form>
{% endblock %}
1 Answer

sekrantz
10,469 PointsI just ran into this problem as well.
It seems the task writeup fails to mention the articles:detail
url requires pk=articles.pk
, and you also have to specify the success_url
field for the ArticleDelete
class.
Here's my passing template:
<h1>{{ article.title }}</h1>
<form method="POST">
{% csrf_token %}
<input type="submit" value="Delete">
<a href="{% url 'articles:detail' pk=article.pk %}">Cancel</a>
</form>
and the DeleteView:
from django.core.urlresolvers import reverse_lazy
### other stuff
class ArticleDelete(DeleteView):
model = models.Article
success_url = reverse_lazy('articles:detail')