Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial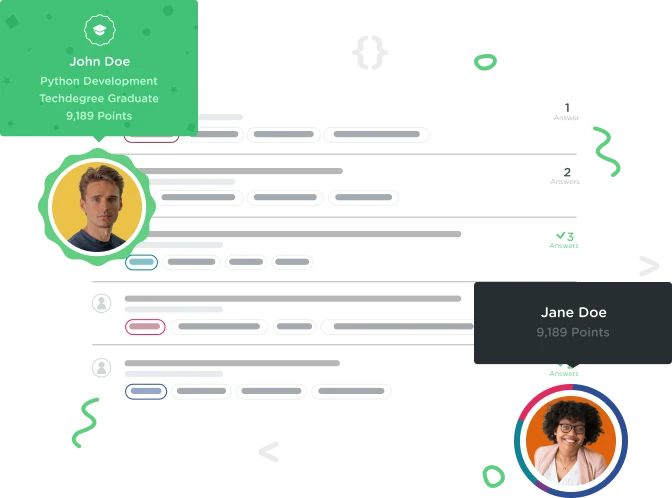

Marco Toniato
9,422 PointsWhy does this happen?
<?php
define("USE_FULL-NAME", FALSE);
define("MAX_BADGES", 150000);
$first_name = "Hampton";
$last_name = "Paulk";
$location = "Orlando, FL";
$role = "Teacher";
$name = $first_name;
if("USE_FULL_NAME" == TRUE){
$name = $first_name . " " . $last_name;
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8>
<title><?php echo $name ?> | Treehouse Profile</title>
<link href="css/style.css" rel="stylesheet" />
</head>
<body>
<section class="sidebar text-center">
<div class="avatar">
<img src="img/avatar.png" alt="<?php echo $name ?>">
</div>
<h1><?php echo $name ?></h1>
<p><?php echo $location ?></p>
<hr />
<p>Welcome to PHP Basics!</p>
<hr />
<ul class="social">
<li><a href=""><span class="icon twitter"></span></a></li>
</ul>
</section>
<section class="main">
</section>
</body>
</html>
When Hampton explained about else statements, he said that the else was to prevent $name from running on line 14, updating the value. In the code I pasted above, I did something different. As you can see, I switched the order of the two so that $name would be equals to $first_name first, and then get updated in line 14 if the condition is true, thus avoiding the "else" statement.
Here, however, something weird happens:
if the condition is == TRUE, like in this case, even though I think the code in the "if" statement in line 14 SHOULDN'T run, it runs, and the result is still "Hampton Paulk". And it remains "Hampton Paulk" no matter if the condition is set to TRUE or FALSE.
If the condition is ===TRUE, the opposite occurs. It doesn't matter whether you define "USE_FULL_NAME" as TRUE or FALSE, the condition in line 14 NEVER runs, and the name is just "Hampton" in both cases.
if I set the condition == FALSE and set the USE_FULL_NAME to TRUE, the program works as intended. It displays the first name "Hampton" only. But if I set USE_FULL_NAME to FALSE, the condition should be met and it should display "Hampton Paulk", yet it doesn't, and it only displays "Hampton" regardless.
Why does this happen? What am I getting wrong in the order of operation?
2 Answers

Wojciech Cupa
13,521 PointsHi Marco, Did you noticed that your global variable is different: USE_FULL-NAME (hyphen '-') than global which was checked in the conditional statement: USE_FULL_NAME (underscore '_')?
It could be an explanation why this doesn't work as expected. Cheers!
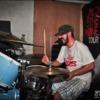
Mike Costa
Courses Plus Student 26,362 PointsWojciech has a good point about being consistent with your var names. "USE_FULL-NAME" if your constant and your conditional uses "USE_FULL_NAME". However, that is not the reason why you are getting unexpected results. You're comparing a string to equal true. You can use ANY string equal to a truthful boolean and it will equal true. Examples would be ("Marco" == true), ("Wojciech" == true), ect. Being that you are using a constant, you don't need to put quotes around the var name. if you did it like this:
define("USE_FULL_NAME", FALSE);
if(USE_FULL_NAME == true){
$name = $first_name . " " . $last_name;
}
You'll be good to go!