Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial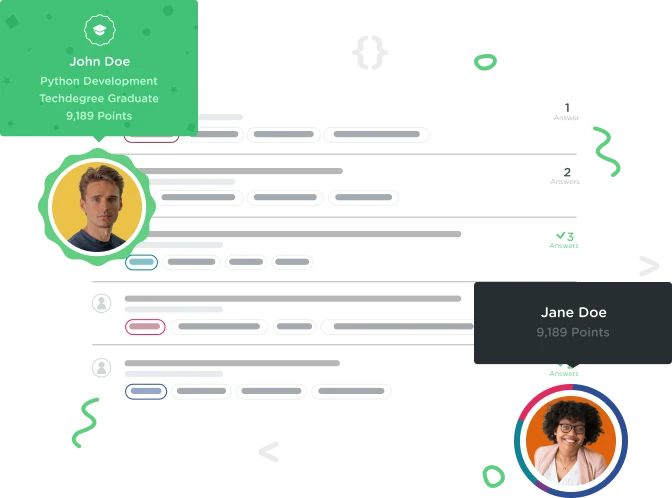

Siddharth Pandey
7,280 PointsWhy does this not work for creating a save state?
At the end of the video Guil gave us a challenge of creating a save state here is my code by I get the syntax error: "error unexpected token )" whenever I attempt to run this code why is this?
const form = document.querySelector('#registrar');
const input = form.querySelector('input');
const ul = document.querySelector('#invitedList');
function createLI(text) {
const li = document.createElement('li');
const span = document.createElement('span');
span.textContent = text;
li.appendChild(span);
const label = document.createElement('label');
label.textContent ='Confirmed';
const checkbox = document.createElement('input');
checkbox.type = 'checkbox';
label.appendChild(checkbox);
li.appendChild(label);
const editButton = document.createElement('button');
editButton.textContent = 'Edit';
li.appendChild(editButton);
const removeButton = document.createElement('button');
removeButton.textContent = 'Remove';
li.appendChild(removeButton);
return li;
}
form.addEventListener('submit', function submit(name) {
name.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text);
ul.appendChild(li);
});
ul.addEventListener('change', function styling(e) {
const checkbox = e.target;
const checked = e.target.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = 'responded';
} else {
listItem.className = '';
}
});
ul.addEventListener('click', function remove(e) {
const listItem = e.target.parentNode;
const ul = listItem.parentNode;
const button = e.target;
const li = button.parentNode;
if (button.tagName == 'BUTTON') {
if (button.textContent === 'Remove') {
ul.removeChild(listItem);
} else if (button.textContent === 'Edit') {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'Save';
} else if (button.textContent === 'Save') {
createLI(input);
}
});
1 Answer
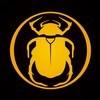
rydavim
18,813 PointsIt looks like there is a secondary problem with your Save button, but to solve the current issue - you're just missing a closing }
on your if
statement in the last section.
ul.addEventListener('click', function remove(e) {
const listItem = e.target.parentNode;
const ul = listItem.parentNode;
const button = e.target;
const li = button.parentNode;
if (button.tagName == 'BUTTON') {
if (button.textContent === 'Remove') {
ul.removeChild(listItem);
} else if (button.textContent === 'Edit') {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'Save';
} else if (button.textContent === 'Save') {
createLI(input);
}
} // CLOSING BRACE GOES HERE
});
That'll fix the error and you can then work on the Save. Let me know if you have any follow up questions. Good luck, and happy coding!
Siddharth Pandey
7,280 PointsSiddharth Pandey
7,280 PointsGuill explained how to make the save button in the next video. However, when I attempted it again nothing was actually saving when I pressed save, no text appeared, can you please tell me what is wrong with my code.
Thanks for the help! Sid
rydavim
18,813 Pointsrydavim
18,813 PointsYou've got a typo where you forgot to camelCase
textContent
.Should work if you fix that - your code looks good.
console.log
statements are really helpful for working out these kinds of problems. If you put one after that line, you can see that the content is empty.Happy coding!