Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial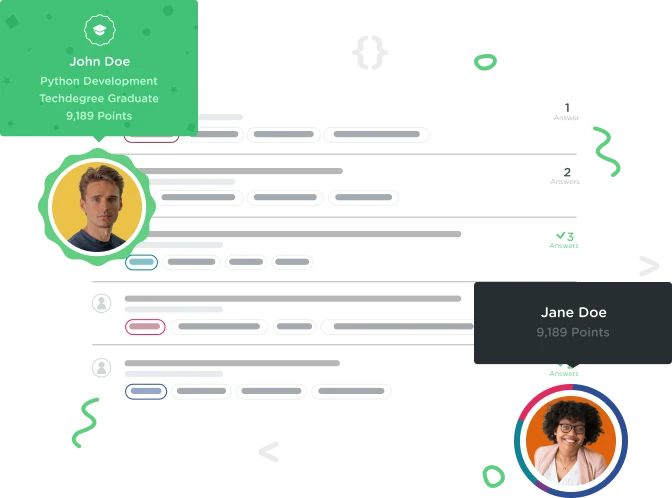
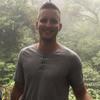
Max Green
12,516 PointsWhy does this only falter when there's two vowels in a row?
The generated combination of letters always seems to have two vowels paired next to each other. This is what's stumping me. For some reason my code doesn't catch the 2nd vowel in any pair of vowels. Help :S. I've been on this for a stupidly long amount of time.
def disemvowel(word):
new_list = list(word)
for letter in new_list:
if letter.upper() == "A":
new_list.remove(letter)
continue
elif letter.upper() == "E":
new_list.remove(letter)
continue
elif letter.upper() == "I":
new_list.remove(letter)
continue
elif letter.upper() == "O":
new_list.remove(letter)
continue
elif letter.upper() == "U":
new_list.remove(letter)
continue
newest_list = "".join(new_list)
return newest_list
1 Answer
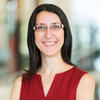
Louise St. Germain
19,424 PointsHi Max,
Looks like as the for loop steps through your list, you're also modifying that very same list, so Python's indexing as it steps through the list gets out of sync when you start deleting elements on the fly. For example, say:
new_list = ['a', 'A', 'b', 'c']
The first "a" is at index 0, the second one "A" is at 1, and so on up to c at index = 3.
Python hits on the first "a" (index location 0) and it gets deleted. So now that the first letter is gone, the updated list is ['A', 'b','c'], with "A" now sitting at index 0, b at 1 (was 2 before), and c at 2 (was 3 before).
THEN Python says, hey, I've finished everything at index location 0 (which, as it turns out, has just been updated to a letter you haven't checked yet), and steps to index location 1 (which is now 'b'). It ends up skipping over that second A entirely - doesn't even evaluate it.
Your best bet would probably be to build your output in another variable as you go along, i.e., don't delete anything out of your original list, but just add a condition that says that if the letter is a consonant, add it to your new variable as you step through the original list (and if it's a vowel, don't add it). That way the indexing on the original list won't get messed up and it will evaluate every single letter.
Good luck! :-)
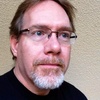
Chris Freeman
Treehouse Moderator 68,441 PointsChanged comment to answer.
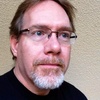
Chris Freeman
Treehouse Moderator 68,441 PointsMax, you could also use the slice notation to create a copy of new_list in the for
loop statement. Deletions from new_list
would no longer affect the for
loop. Notice the [:] added to the end of new_list
:
for letter in new_list[:]:
In, general, it is never a good practice to modify the container that you are looping over in a for
loop.
Max Green
12,516 PointsMax Green
12,516 PointsAhhh yea I get it now. I never thought of building another word. Very clever :)