Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial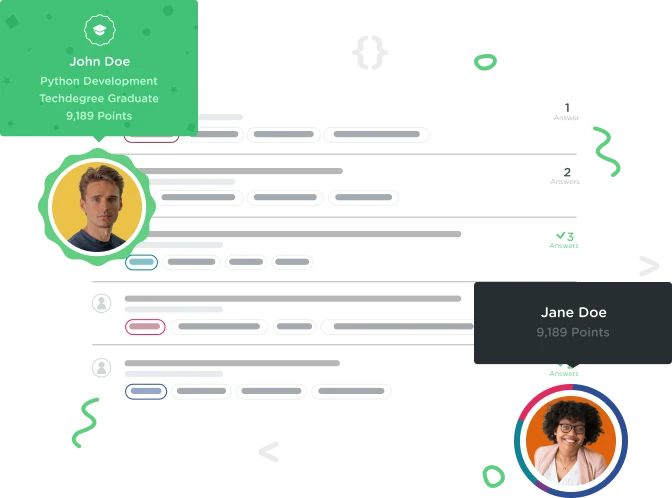
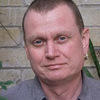
Unsubscribed User
4,479 PointsWhy does this version of a 'do, while' number guessing game not work?
While revising the 'do, while' loop video and exercise, I tried this, but it doesn't work.
I think it generates a condition that will always evaluate 'true', but can someone clarify this more?
Is there anything wrong with generating a random number (1 - 10) and storing it in a variable in this way?
var randomNum = Math.floor(Math.random() * 10 + 1);
do {
var guess = prompt("Guess a number between 1 and 10.");
parseInt(guess);
} while ( guess !== randomNum )
document.write("you guessed the random number is " + randomNum);
4 Answers

Jon Mirow
9,864 PointsHmm, that code seems to work for me. I added an alert so I'd know what number it had, and ran it a few times without error:
var randomNum = Math.floor(Math.random() * 10 + 1);
do {
alert(randomNum)
var guess = parseInt(prompt("Guess a number between 1 and 10."));
} while ( guess !== randomNum )
document.write("you guessed the random number is " + randomNum);

Jon Mirow
9,864 Pointsho there!
parseInt returns the value, it doesn't change the variable in place, so the guess is always a string, so the condition is always false.
for example:
name = prompt("What's your name?")
alert(name)
parseInt(name)
alert(name)
name = parseInt(name)
alert(name)
Only once the returned value is assigned to the variable does name change, so name becomes NaN because something like "Bob" isn't a number, so that's what parseInt() returns.
If you used this comparison:
while ( guess != randomNum )
You also wouldn't get the infinite loop, because == will attempt type conversion (though it's better to parseInt and use === usually)
Hope it helps :)
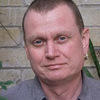
Unsubscribed User
4,479 PointsYou're right - it does work.
When I first tested the code I kept entering the same number, thinking that 'var randonNum' was generating a new random number each time. But testing it again, I entered 1 then 2, and up to 10 if necessary, before I got the single random number value stored in 'randomNum'.
Thanks for looking at this Jon.

Jon Mirow
9,864 PointsNo worries!
Mistakes are a good thing - just yesterday I spent 20 minutes trying to work out why a test I'd put in my code wasn't working, then remembered I'd commented out it's output lol.
It's the code that works first time you've really gotta be scared of lol
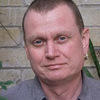
Unsubscribed User
4,479 PointsThis was an earlier attempt that I think addresses the parsing and storing of the number value in 'guess', but it is still not working ?
var randomNum = Math.floor(Math.random() * 10 + 1);
do {
var guess = parseInt(prompt("Guess a number between 1 and 10."));
} while ( guess !== randomNum )
document.write("you guessed the random number is " + randomNum);