Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial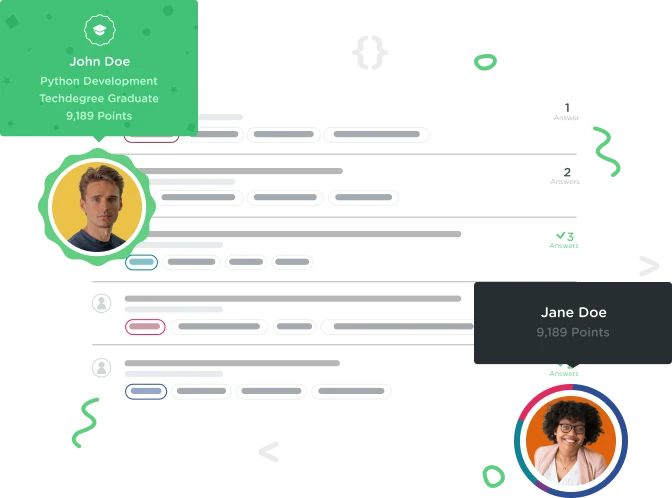
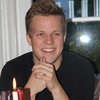
William Larsten
UX Design Techdegree Graduate 17,195 PointsWhy does this work in Xcode, but not in the challenge?
This is the code I've written in the code challenge to assign the variable result with the tuple from the function and pass it the value "Tom". I seem to get it to work in Xcode and get what I think is the right result, but I keep getting an error message in the code challenge. Where's my thinking wrong? Is the code challenge looking for something else or another type of solution?
func greeting(person: String) -> (language: String, greeting: String) {
let hello = ("English", "Hello \(person)")
return hello
}
var result = greeting("Tom")
println(result)
4 Answers
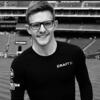
agreatdaytocode
24,757 PointsTry this:
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting("Tom")
Notice the first line
"language: String, greeting: String" vs "greeting: String, language: String"
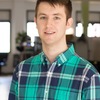
Ethan Lowry
Courses Plus Student 7,323 PointsWhile your code seems valid, it could just be the challenge being overly picky, which sadly happens sometimes. Try swapping the order of the elements in your returned tuple, i.e. greeting first, then language, the way it was described in an earlier stage when you first set the return type to be a tuple. Remember to change where you actually return the tuple accordingly, as well.
Hopefully that's all the problem is, I'm pretty sure I helped someone else recently on this challenge who ran into this issue as well.
(Also just in case, in the originally provided code the constant inside the function is called "greeting", whereas you've changed it to "hello". I recommend not messing around with the given code when it's not necessary as it can occasionally break the challenges.)

Chris Shaw
26,676 PointsHi William,
The challenge isn't expecting the code you have above, instead it's still expecting the constants that were predefined in the challenge itself for task 1 so instead you would want the below.
func greeting(person: String) -> (language: String, greeting: String) {
let language = "English"
let greeting = "Hello \(person)"
return (language, greeting)
}
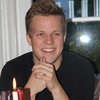
William Larsten
UX Design Techdegree Graduate 17,195 PointsGreat answers Chris and Ethan. I've tried the following code based on your suggestions and I gotten cleaner code that still works in Xcode and is closer to what I think they want. But I still can't get past the challenge.
func greeting(person: String) -> (language: String, greeting: String) {
let language = "English"
let greeting = "Hello \(person)"
return (language, greeting)
}
var result = greeting("Tom")
The error message says I have the wrong value for the result variable which is supposed to contain the tuple returned from the greeting function. But when I reference what the result variable contains in Xcode it is (.0 "English",.1 "Hello Tom") which is a correct tuple if I'm not completely mistaken?