Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial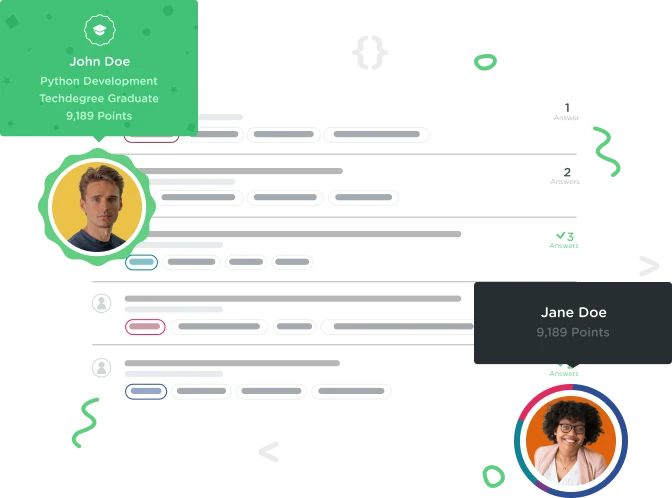
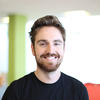
Kristian Woods
23,414 PointsWhy does "undefined" keep showing?
Can't see why "undefined" keeps appearing...
can anyone help?
var showQuiz = [
["Where does Jon Snow appear?", "Game Of Thrones"],
["Where does Eric Foreman appear?", "That 70s Show"],
["Where does Luke Skywalker appear?", "Star Wars"]
];
var answer;
var question;
var response;
var correct = [];
var wrong = [];
var html;
function print(message) {
var results = document.getElementById("results");
results.innerHTML = message;
}
for(var i = 0; i < showQuiz.length; i++) {
question = showQuiz[i][0];
answer = showQuiz[i][1];
response = prompt(question);
if(response === answer) {
correct.push(question);
} else {
wrong.push(question);
}
}
function results(arr) {
var listHTML = "<ol>";
for(var i = 0; i < arr.length; i++) {
listHTML += "<li>" + arr[i] + "</li>";
}
listHTML += "</ol>";
return listHTML;
}
html += "<h2>You got thse questions correct:</h2>";
html += results(correct);
html += "<h2>You got thse questions incorrect:</h2>";
html += results(wrong);
print(html);
1 Answer
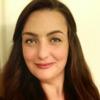
Jennifer Nordell
Treehouse TeacherHi there! It's because of the underlying value of this variable:
var html;
Because you didn't initialize it, it starts with a value of undefined. But it's an easy fix!
var html = " ";
Now it's an empty string and will be appended to as you expect. Hope this helps!
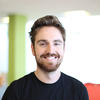
Kristian Woods
23,414 PointsThank you. But why is that the case? I thought you could leave a variable undefined at the beginning, as long as you define it at some point?
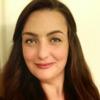
Jennifer Nordell
Treehouse TeacherKristian Woods That's just the way JavaScript works. When you define a variable, but don't give it an initial value JavaScript automatically assigns it a primitive data type to start it off with. So it assigns it undefined
.
Let's put it this way. You declared the variable html
which now has a value of undefined. And then you started appending on that. So your code did exactly what you said it should do. It printed undefined
(which was the value) and then your <h2>
elements and strings.
It is generally considered best practice to initialize any variable you know will hold a string with a string so that there's no ambiguity later on. And while I'm not a big fan of this site, initialization is mentioned among best practices here:
http://www.w3schools.com/js/js_best_practices.asp
Here's some documentation from MDN about the creating of global objects
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/undefined
Hope this clarifies some things!
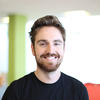
Kristian Woods
23,414 PointsThank you for clearing that up. Really appreciate it
Trevor Covington
9,050 PointsTrevor Covington
9,050 PointsIn your print function, you are using getElementById() to retrieve an element with an id of results. Since there are no elements with that id in this code, it is returning null. You can do something like this:
That creates a div and adds an id of "results" to it.