Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial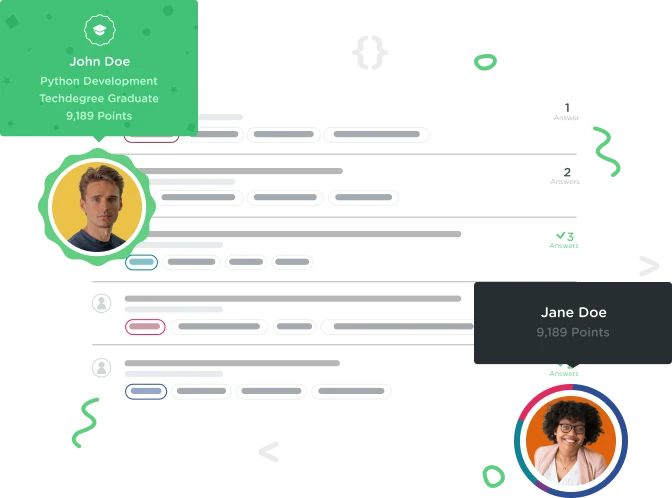
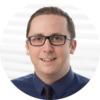
Andrew Keith
Full Stack JavaScript Techdegree Graduate 19,728 PointsWhy doesn't arrow functions work correctly with "this"?
I approached it like this: const myString = { string: "Programming with Treehouse is fun!", countWords: () => { return this.string.split(' ').length; } } but node returned the error "Cannot read property 'split' of undefined". If I console.log "this" within my method I get an empty object. Is there something I'm missing? I thought arrow functions were just a shorthand for function(){}.
1 Answer
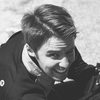
Brandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsHello Andrew,
I too thought arrow functions were shorthand for function() {} when I was first introduced to them. But as it turns out, they do add some special functionality. Specifically when it comes to "this."
This is a relatively big topic, so I won't go into crazy depth here. However I'll provide some additional resources that will hopefully get you on the right path.
First of all, your code. Try removing the arrow function from:
countWords: () => {
return this.string.split(" ").length;
}
so it looks like this:
countWords: function() {
return this.string.split(" ").length;
}
So the question is, why does "this" return return an empty object instead of the myString object?
The short answer is that since you're using an arrow function, "this" is bound to the countWords function/method instead of the myObject object.
This is the first article I'd suggestion reading. Specifically the Main benefit: No binding of βthisβ section towards the bottom of the article. Here's an excerpt:
In classic function expressions, the this keyword is bound to different values based on the context in which it is called. With arrow functions however, this is lexically bound. It means that it uses this from the code that contains the arrow > function.
"this" is a very confusing and misunderstood aspect of JavaScript. If you want to go deeper into how it works, Kyle Simpson has written a book (available for free on GitHub) that goes deep into how "this" works.
There are four rules to determine the context of "this":
- Is the function called with new (new binding)? If so, this is the newly constructed object.
- Is the function called with call or apply (explicit binding), even hidden inside a bind hard binding? If so, this is the explicitly specified object.
- Is the function called with a context (implicit binding), otherwise known as an owning or containing object? If so, this is that context object.
- Otherwise, default the this (default binding). If in strict mode, pick undefined, otherwise pick the global object.
Treehouse also has a workshop on arrow functions that I'd recommend checking out if you haven't already done so.
I hope this helps! If you have any additional questions, please let me/us know!
Have a great weekend, Brandon