Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial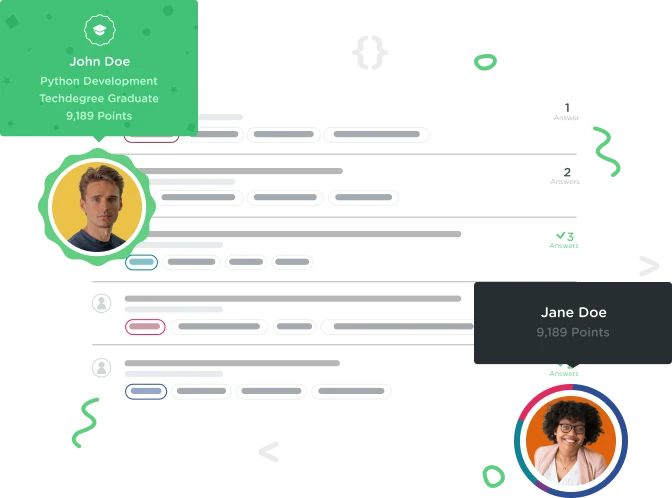

Felix Putra
Python Web Development Techdegree Student 1,927 PointsWhy doesn't my code remove the vowel letters?
Hi guys,
I've tried to make my own code, and here is the result. My code doesn't remove any of the vowel letters. In fact, it prints out the same thing as the variable letters_list. Any help will be very appreciated and thank you in advance!
2 Answers
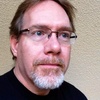
Chris Freeman
Treehouse Moderator 68,423 PointsI found the following issues with your code.
- A
list()
of alist
returns the same list. That is:
>>> letters = ["abcde"]
>>> chunked = list(letters)
>>> letters
['abcde']
>>> chunked
['abcde']
>>> letters == chunked
True
If you wanted chunked
to be ['a', 'b', 'c', ...]
either start with letters equal to just the string "abcdefghijklmnopqrstuvwxyz" or set chunked = list(letters_list[0])
in the
if/elif/else
statement, the conditions should be using the called method.lower()
(with parens). Otherwise, you are comparing the existence of a method called 'lower` with a string. This will always fail.You do not have a way to increment your index
i
At the end of the loop, the
length
is set to-1
. This will fail thewhile
condition on the first iteration. You probably wantedlength -= 1
to decrement the length.
After correcting the above and rerunning:
- printing
chunked[i]
at the end of the loop will print the current index even if it has been deleted. When the 'a' is deleted in the first iteration withi=0
, theprint(chunked[i])
will print 'b'
Good Luck!

Bob Buethe
3,276 PointsAs Chris said, list(letters_list) will just return the same string. You need to use list(letters_list[0])
Let's step through this:
Start:
chunked = ['a', 'b', 'c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
length = 25
i = 0
FIRST PASS:
'a' is removed, so chunked = [ 'b', 'c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
length is set to -1 (because the statement reads "length =- 1" instead of "length -= 1")
i is still 0
Since length is now less than 0, the procedure ends.
But what happens if "length =- 1" is changed to "length -= 1"? In that case:
FIRST PASS:
'a' is removed, so chunked = [ 'b', 'c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
length is set to 24 i is still 0
SECOND PASS:
chunked[0] is 'b', so nothing is changed.
length is set to 23
i is still 0
THIRD PASS:
chunked[0] is still 'b', so nothing is changed.
length is set to 22
i is still 0
And so on, until length = -1. You're decrementing length, but not incrementing i, so the program keeps looking at chunked[0] at every pass.
But these loops aren't necessary, because remove will find the letter to remove wherever it is in the list. So all you need to do is:
from string import join
letters_list = ["abcdefghijklmnopqrstuvwxyz"]
chunked = list(letters_list[0])
chunked.remove('a').remove('e').remove('i').remove('o').remove('u')
print(join(chunked, '')