Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial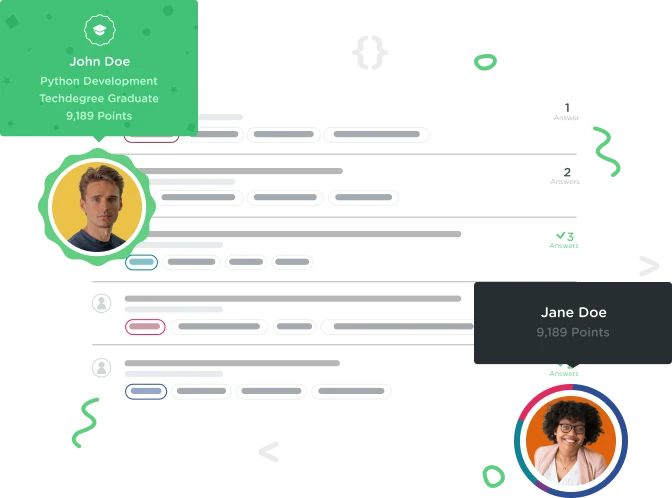
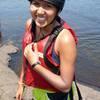
neilatoofuny
2,362 Pointswhy doesn't my code work?
- Create a function named nchoices() that takes an iterable and an integer. The function should return a list of nrandom items from the iterable where n is the integer. Duplicates are allowed.
my code:
def nchoices(iterable, n):
n_list = []
number = int(n)
# get the random item from the list
#put n random items in the list
while number > 0:
random_item = random.choice(iterable)
n_list.append(random_item)
number -=1
#return the list
return n_list
2 Answers

Jeff Morrison
5,917 PointsWhat output are you getting, which you weren't expecting? From looking at your code:
- You need to import random in order to use it (which maybe you did and didn't copy and paste)
- Your while and return should be indented - otherwise they're not part of the function
- Instead of a while loop and decrementing (count n down) - I would use the range() function, which does the work for you. This is built in to do loop management for you.
import random
def nchoices(iterable, n):
n_list = []
for _ in range(n):
##do the choosing and appending here
return n_list
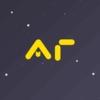
AR Ehsan
7,912 PointsHere how I did it
import random
def nchoices(iterable, count):
lst = []
for x in range(count):
lst.append(random.choice(iterable))
return lst