Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial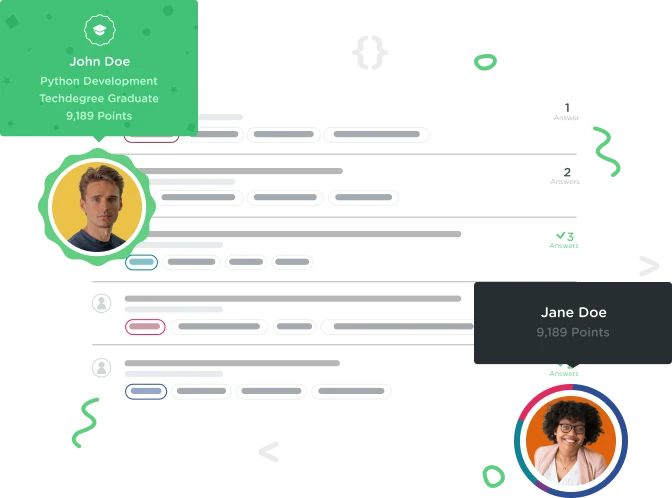

Nate Mulinga
809 PointsWhy Doesn't My Code Work?: Returning Complex Values In Swift Functions
Why Doesnt My Code Work? I don't understand why I can't return values lat and lon in the function:
func getTowerCoordinates (place: String) -> (var lat:Double, lon: Double){
switch place {
case "Eiffel Tower" : lat: 48.8582, lon: 2.2945
case "Great Pyramid" : lat: 29.9792, lon: 31.1344
case "Sydney Opera House" : lat: 33.8587, lon: 151.2140
default: print("Who cares")
}
return (lat, lon)
}
5 Answers

Greg Kaleka
39,021 PointsHi Nate,
There are a few things wrong with your code, but you're actually pretty close.
- When you declare a function, you only specify the return type - you're trying to declare variables at the same time. Change the return to
(Double, Double)
, since that's the type: a tuple with two Doubles. - Now we need to declare the constants
lat
andlon
inside the function (e.g.let lat: Double
) - In your switch statements, you've got a bunch of extraneous colons. What you should be doing is for each case, setting the value of the constants
lat
andlon
. For example, case "Eiffel Tower" : [new line] lat = 48.8582 [new line] lon = 2.2945 - The instructions tell you what to return in the default case. If you don't follow those instructions, you'll also get a compiler error, because you're trying to use values that may not have been initialized in the return statement.
I'm not going to post a full solution, because I think you'll benefit from making the fixes on your own. Post back here when you've gotten it to work!
Cheers
-Greg

Nate Mulinga
809 PointsThanks so much! Your answer helped me out a bunch:) Heres what I have....
func getTowerCoordinates(location: String) -> (lat: Double, lon: Double) {
var lat: Double = 0.0, lon: Double = 0.0
switch location {
case "Eiffel Tower": lat = 48.8582; lon = 2.2945
case "Great Pyramid": lat = 29.9792; lon = 31.1344
case "Sydney Opera House": lat = 33.8587; lon = 151.2140
default: return (0,0)
}
return (lat, lon)
}

Greg Kaleka
39,021 PointsYou're super close - you just need to make one more tweak: Your return type is a named tuple, but the challenge wants an unnamed tuple; just remove the lat: and lon: from it.

Anthia Tillbury
3,388 PointsI was on this problem for a few days, on and off and couldn't do it, this is as close as I got, it doesn't look to different to the post above actually?
func getTowerCoordinates(location location: String) -> (Double, Double) {
let lat: Double
let lon: Double
switch location {
case "Eiffel Tower": let lat = "48.8582"; let lon = "2.2945"
case "Great Pyramid": let lat = "29.9792"; let lon = "31.1344"
case "Sydney Opera House": let lat = "33.8587"; let lon = "151.2140"
default: (0,0)
}
return (lat, lon)
}
getTowerCoordinates(location: Eiffel Tower)
I changed it to the following based on the directions in the reply from Greg, but still cannot pass in the value in from outside the function:
func getTowerCoordinates(location location: String) -> (Double, Double) {
var lat: Double
var lon: Double
switch location {
case "Eiffel Tower":
var lat = 48.8582
var lon = 2.2945
case "Great Pyramid":
var lat = 29.9792
var lon = 31.1344
case "Sydney Opera House":
var lat = 33.8587
var lon = 151.2140
default: (0.00,0.00)
}
return (lat, lon)
}
getTowerCoordinates(location: Eiffel Tower)

Greg Kaleka
39,021 PointsHey James,
A few issues.
1) The code challenge doesn't want a labeled parameter, so remove that:
func getTowerCoordinates(location: String) -> (Double, Double) {
2) You are re-declaring your variables lat
and lon
in the switch statement - don't do that!
switch location {
case "Eiffel Tower":
lat = 48.8582
lon = 2.2945
// etc.
3) Your default case doesn't do anything - you should instead set lat and lon to 0 and 0. You could return (0.0, 0.0), but if you do that, you need to initialize lat and lon when you declare them, since you're trying to return lat and lon later, and it may not be initialized yet. In short - you should probably just set them to 0 in the default case.
default:
lat = 0.0
lon = 0.0
4) You need to pass in a String.
// note no label since we removed it above
getTowerCoordinates("Eiffel Tower")

Anthia Tillbury
3,388 PointsHi Greg, many thanks for checking my work!
Initially I didn't have a label on the parameter, I think that after all of the combinations I tried it must have slipped in.
Re-declaring the variables seems ridiculous, I don't know why I did that!
The reason I used "0.0" was because it was a Double, I thought that it would have to have something after the decimal place, unlike Int, I guess I was wrong. I'll remember that about "0", I've been reading over "Initialisation" but it's a little bit of a mind bender for me right now; https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Initialization.html

Greg Kaleka
39,021 PointsSorry for the confusion - nothing to do with 0 vs 0.0. All I meant is that if your switch statement ended up in the default case you would never have given lat or lon a value.
As for initialization: it just means giving your variables a value. We can either do this at the time of declaration or later on, but we must do it before using the variables.
//example 1
var lat: Double // just declaration; no initialization
lat = 25.5 // initialization
//example 2
var lon: Double = 0.0 // declaration and initialization
Hopefully that makes more sense

Anthia Tillbury
3,388 PointsUnderstood. Thank you for taking the extra time!
I used quotation marks to pass in the value to the function, but then ran into the error you were referring too: initialisation as the compiler warned me that I was trying to attain a value without it being initialised and the example above gave it away: I needed to initialise the variables "lat" and "lon" when declared inside the function and not it works!

Greg Kaleka
39,021 PointsHopefully your last sentence was meant to say "now it works"
If so - yay! Glad I was able to clear things up for you.
Cheers
-Greg