Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial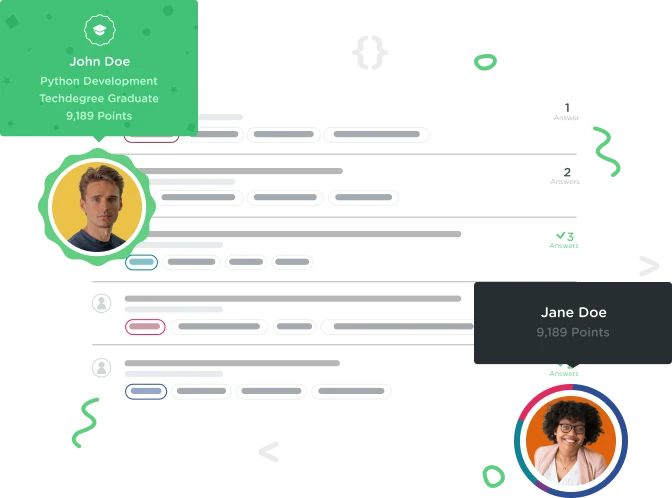
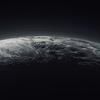
Robert Harmon
1,464 PointsWhy doesn't my FizzBuzz solution work?
I'm returning an error that says "binary operator '~=' cannot be applied to operands of type 'Bool' and 'Int'" on each of my "case" lines. What does that mean?
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case (n % 3 == 0) && (n % 5 == 0): return "FizzBuzz"
case n % 3 == 0: return "Fizz"
case n % 5 == 0: return "Buzz"
}
// End code
return "\(n)"
}
4 Answers
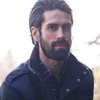
Josh Dobson
3,127 Pointstake this example and put it into your xcode playground.
it's going to show an error on the second case of "expression pattern of type Bool cannot match values of type 'Int"
It's saying the value you are giving it num
is an Int
but what the switch is checking for is a Bool
which is not allowed. Like in ghostbusters...you can't cross the streams! The switch needs to check for the type of value you are giving it with num
good luck!
let numbers = [1,2,3,4,5,6,7,8,9]
for num in numbers {
switch num {
case 1: print("1: \(num)")
case (num == 1): print("1: \(num)")
default: print(num)
}
}
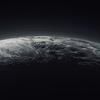
Robert Harmon
1,464 PointsI see. Thank you Josh!
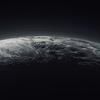
Robert Harmon
1,464 PointsThanks for your help, Josh. Unfortunately I'm still confused. I don't think I fully understand the fundamental difference between the workings of an "if" statement and a "switch" that you're hitting on.
Does an if statement like the one I've listed below not also look to "match on true or false" values?
Thanks again, and please forgive my ignorance. This is my first foray into any kind of programming.
if (n % 3 == 0 ) && (n % 5 == 0) { return "FizzBuzz" } else if (n % 3 == 0 ){ return "Fizz" } else if (n % 5 == 0) { return "Buzz" }
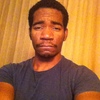
Stephen Whitfield
16,771 PointsYou're conditionals should be implemented using if/else statements, not switch statements.
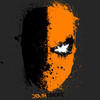
Jacob Boyd
1,925 PointsYou could use case let _ where
syntax to use a switch statement here
Josh Dobson
3,127 PointsJosh Dobson
3,127 PointsRight now it appears each case is looking to match on true or false values which
n
will never you give you.n
never actually gets run through any case.A switch isn't the best solution for this problem since we are wanting to ask "does the current number
n
match these statements" I would think we would have to have a case for every number if we wanted to use a switch.I would try something with if and else if instead.