Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial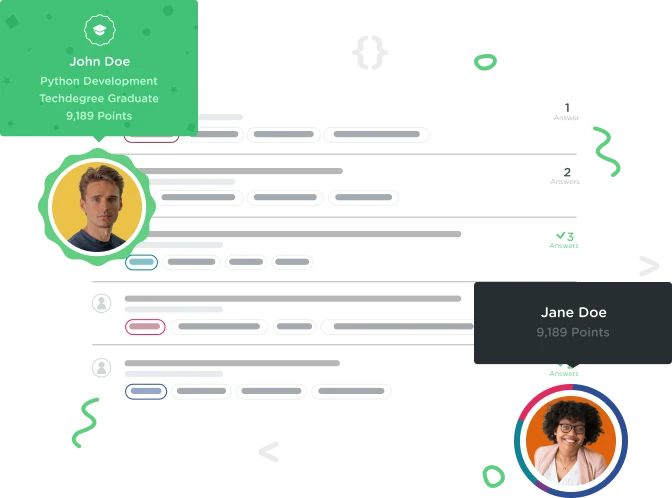
4 Answers
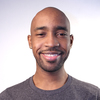
David Tonge
Courses Plus Student 45,640 PointsI didn't run it but there's a missing semi-colon after the second alert function. See if that changes anything.

Simon Coates
28,694 PointsI think you have a problem with { and }. Remember to indent so you can see how { and } match up. You also have:
1) excessive use of var. Within a scope, i think you only need to use it when you first create the variable.
2) rank = rank + 0; is pointless.
3) spelling mistake (you refer to a variable called 'fiftht') will lead to a null problem.
4) if you don't use the guess variable, then it serves no purpose.
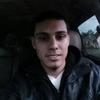
jose macedo
6,041 Pointsi dont see it
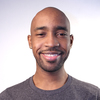
David Tonge
Courses Plus Student 45,640 PointsI'm not at my computer, so I can't run your code but paste your code into http://www.jslint.com/ ... there seems to be some missing semi-colons and other errors.

Simon Coates
28,694 PointsThe following might be a little near to what you want. I fixed some stuff and got rid of lines that didn't do anything, or where nothing used the variable in the script:
// intro
var rank = 0;
var name = prompt('what is your name?');
alert('hello ' + name + ' this quiz will test your knowledge on life skills');
alert('only type in your answer as a letter');
// question 1
var first = prompt('when you shake a hand, should you A.shake soflty B.shake firmly with a grip C.shake fast high-up and low-down?');
if(first.toUpperCase() === 'B' ) {
rank = rank + 1;
alert("that's correct");
} else if (first.toUpperCase() === 'C' ) {
alert('you slow!');
} else if (first.toUpperCase() === 'A' ) {
alert('not right!');
} else {
var firsttry = prompt('you need to type your answer as a letter!, try again.when you shake a hand, should you A.shake soflty B.shake firmly with a grip C.shake fast high-up and low-down?')
if(firsttry.toUpperCase() === 'B' ) {
rank = rank + 1;
alert("that's correct");
} else if (firsttry.toUpperCase() === 'C' ) {
alert('you slow!');
} else if (firsttry.toUpperCase() === 'A' ) {
alert('not right!');
}
}
//question 2
var second = prompt('how many Gs are in a oz.A 12. B 22. C 28 you have ' + rank + ' answers correct');
if(second.toUpperCase() === 'C' ) {
rank = rank + 1;
alert("that's correct");
} else if (second.toUpperCase() === 'B' ) {
alert('you slow!');
} else if (second.toUpperCase() === 'A' ) {
alert('not right!');
} else {
var secondtry = prompt('you need to type your answer as a letter!, try again.how many Gs are in a oz.A 12. B 22. C 28 you have ' + rank + ' answers correct');
if(second.toUpperCase() === 'C' ) {
rank = rank + 1;
alert("that's correct");
} else if (secondtry.toUpperCase() === 'B' ) {
alert('you slow!');
} else if (secondtry.toUpperCase() === 'A' ) {
alert('not right!');
}
}
// question 3
var third = prompt('is red A.positive or B.negative on a battery? you have ' + rank + ' answers correct');
if(third.toUpperCase() === 'A' ) {
rank = rank + 1;
alert("that's correct");
} else if (third.toUpperCase() === 'B' ) {
alert('you slow!');
} else {
var thirdtry = prompt('is red A.positive or B.negative on a battery? you have ' + rank + ' answers correct');
if(thirdtry.toUpperCase() === 'A' ) {
rank = rank + 1;
alert("that's correct");
} else if (thirdtry.toUpperCase() === 'B' ) {
alert('you slow!');
}
}
// question 4
var fourth = prompt('what is 12 multiplied by 12? A.122 B.144 C.84 you have ' + rank + ' answers correct');
if(fourth.toUpperCase() === 'B' ) {
rank = rank + 1;
alert("that's correct");
} else if (fourth.toUpperCase() === 'C' ) {
alert('you suck!');
} else if (fourth.toUpperCase() === 'A' ) {
alert('really?!');
} else {
var fourthtry = prompt('you need to type your answer as a letter!, try again.how many Gs are in a oz.A 12. B 22. C 28 you have ' + rank + ' answers correct');
if(fourthtry.toUpperCase() === 'B' ) {
rank = rank + 1;
alert("that's correct");
}else if (fourth.toUpperCase() === 'C' ) {
alert('you suck!');
} else if (fourth.toUpperCase() === 'A' ) {
alert('really!');
}
}
// question 5
var fifth = prompt('what are channel locks? A.a wrench B. a screwdriver C.pliers you have ' + rank + ' answers correct');
if(fifth.toUpperCase() === 'C' ) {
rank = rank + 1;
alert("that's correct");
} else if (fifth.toUpperCase() === 'Bs' ) {
alert('you suck!');
} else if (fifth.toUpperCase() === 'A' ) {
alert('really?!');
} else {
var fifthtry = prompt('what are channel locks? A.a wrench B. a screwdriver C.pliers you have ' + rank + ' answers correct');
if(fifthtry.toUpperCase() === 'C' ) {
rank = rank + 1;
alert("that's correct");
} else if (fifth.toUpperCase() === 'B' ) {
alert('you suck!');
} else if (fifth.toUpperCase() === 'A' ) {
alert('really!');
}
}
// quiz done and results
alert('you are done');
if (rank === 5) {
alert('you are a golden PRO');
} else if (rank === 3 || rank === 4) {
alert('you are a silver NOVICE');
} else if (rank === 2 || rank == 1) {
alert('you are a bronze LOSER');
}

Simon Coates
28,694 Pointsnb: I wouldn't spend too much time on this. In a real world case, you'd probably write something like this using functions and loops - which eliminate the duplication, and really simplifies debugging.
jose macedo
6,041 Pointsjose macedo
6,041 Pointsstill no but thanks