Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial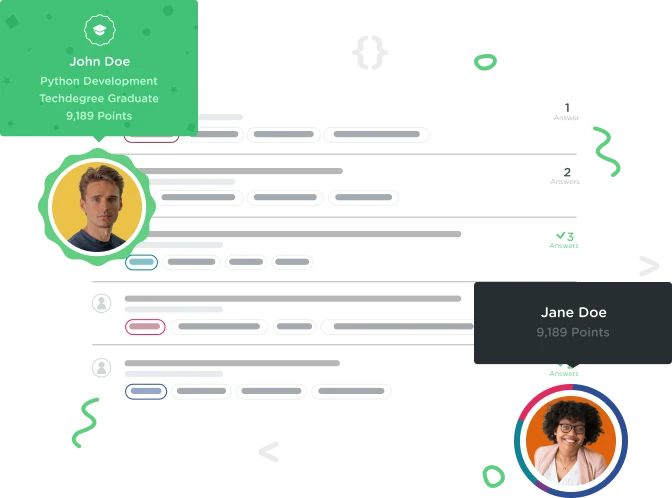

Kevin Murphy
24,380 PointsWhy doesn't the compiler recognize stored property variable declarations in a class/struct initializer?
Why doesn't the compiler recognize num1 in the declaration for num2 below (by default). It will if I lazy load and use the keyword self but I don't understand why it won't otherwise. Note this is a simple example to model the config and session declarations in the Network Operations video.
class Experiment {
var num1: Double = 50.00
var num2: Int = Int(num1) //Error: Experiment.Type does not have a member named 'num1'
}
Why doesn't the compiler recognize num1 inside of the Int initializer for the var declaration of num2?
Thanks.
1 Answer
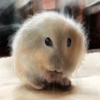
Dominic Pettifer
4,338 PointsI'll attempt to explain this based on my understanding of how Swift works from a book I've read, but someone correct me if I'm wrong.
I think it's because stored properties are initialised before the actual object instance is, thus stored properties can't reference other stored properties on the instance, because the 'instance' (or self) doesn't actually exist yet. As soon as we run init() the instance now exists. I think lazy makes it work because the stored properties get initialised sometime after the object instance is created (i.e. on demand, when first referenced), so now we have a self.
Regarding the error message Experiment.Type does not have a member named 'num1'. Swift thinks the property 'num1' is a static/class property. The following code would fix your issue...
class Experiment {
static var num1: Double = 50.00
var num2: Int = Int(num1)
}
Note the 'static' keyword added to the first property. This means it becomes a stored property of the actual class itself instead of instances of that class. You'd access outside your class like so...
let myNumber = Experiment.num1
Note, we haven't created an instance of Experiment to access num1. I've explained static properties in a bit more detail in answer I gave here https://teamtreehouse.com/forum/what-is-the-reason-for-selfconfig