Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial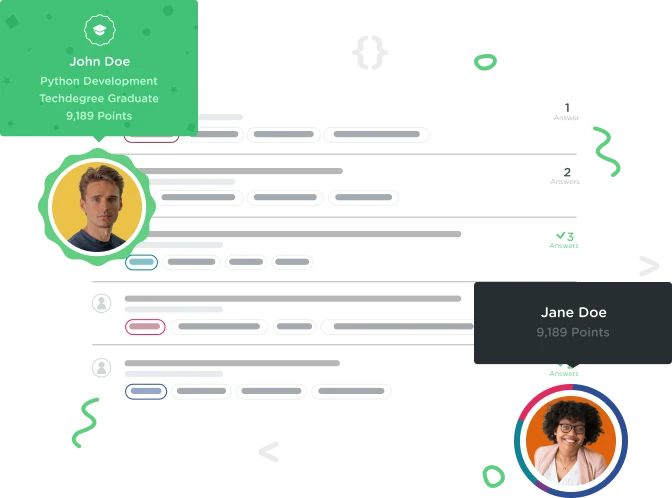
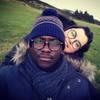
Nathaniel Sem
5,870 PointsWhy doesn't the index value become -1?
Hi Treehouse!
I have been given this problem to solve:
Write a method that returns the "pivot" index of a list of integers.
The pivot index of a list is defined as the index where the sum of the numbers to the left of it, is equal to the sum of the numbers on the right.
Given the list [1, 4, 6, 3, 2], the method should return index 2, since the sum of the numbers to the left of index 2 (1 + 4) is equal to the sum of numbers to the right of index 2 (3 + 2). If no such index exists, it should return -1. If there are multiple pivots, you can return the left-most pivot.
Some more examples:
[ 1, 4, 1, 5, 3, 3 ] would return an index of 3, because the sum of numbers either side of index 3 add up to 6. (1 + 4 + 1 is the same as 3 + 3)
[ 8, 1, 3, 4, 1 ] would return an index of 1, because the sum of numbers either side of index 1 add up to 8. (8 is the same as 3 + 4 + 1)
[ 2, 4, 4, 2, 9 ] would return an index of -1, because there is no index in the array where the sum of numbers to the left of it are equal to the sum of numbers to the right.
So far I have this function:
function seq() {
for(i = 0; i < sequence.length; i += 1) {
if(sequence[i-1] + sequence[i-2] === sequence[i+1] + sequence[i+2]) {
pivot = true;
return sequence.indexOf(sequence[i]);
break;
}else if(pivot === false){
return sequence.indexOf(sequence[i]);
}
}
}
When I ask the console to log sequence[i], it gives me the value "undefined". This is fine however, when I want to display the index of sequence[i] when pivot = false, I get nothing. Should I assume that the index is still -1, or am I doing something wrong here?

Jason Anello
Courses Plus Student 94,610 Pointsfixed code formatting
1 Answer
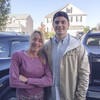
Brodey Newman
10,962 PointsAre you setting pivot to be false by default? Ex: ( let pivot = false; )
Angyal Istvรกn
11,259 PointsAngyal Istvรกn
11,259 PointsHi Nathaniel!
First problem with it, is that, if You start the for loop with i = 0 and sequence's index = i, this means the very first index of the sequence, after in the loop You write i -1 and this isnt exists, beacause the very fisrt index is 0. It makes the problem, but You need to figure out a new loop which do what you want.
Maybe a for loop inside a for loop with if -else can be the right way but i m just guess now.
Some gues for first think, You can start with a loop and check
for(i = 0; i < lenght; i +=1) {
}