Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial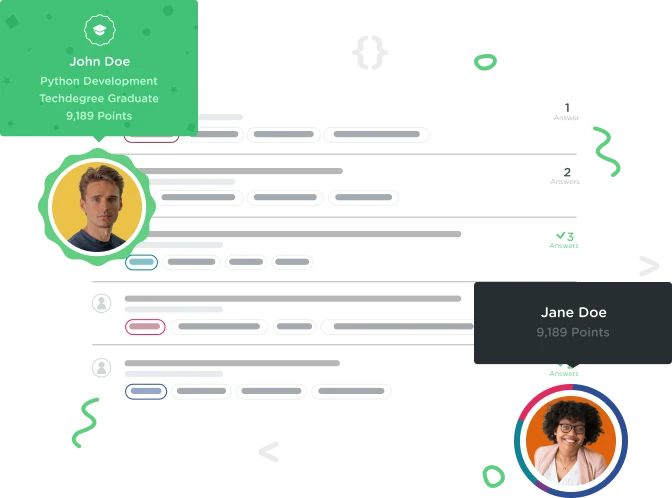

Srikanth Srinivas
1,465 PointsWhy doesn't this code pass?
Im sure that this code functions the way the prompt tells me it should, i checked it myself on an online compiler, yet it doesn't seem to pass. Whys that?
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
anydict = {}
def word_count(anystring):
anystring = anystring.lower()
for word in anystring.split():
anydict[word] = anystring.count(word)
return anydict
1 Answer
Graham Mackenzie
2,747 PointsHey Srikanth!
So, a lot about your code looks good, but a few things need fixing:
- First of all, you need to put your
anydict = {}
declaration within the body of the function. Leaving it outside of the function that returns it could cause technical problems, and is also a bad practice aesthetically / clarity-wise. - You've got the right idea in calling the
.lower()
function on the string that is passed in, but assigning it to itself is also potentially problematic / inelegant. Better to assign it to a whole new variable. - Calling
.split()
as the next step is also the right idea, but you really want to do that outside of the for loop structure, and assign the result to its own new variable as well. - Notice that the instructions say to
check for inclusion in the dict (with "if word in dict"-style syntax). Or add it to the dict...
This is your clue that it's a good idea to use anif... else...
statement here. Obviously, going this direction is going to require you to use a counting variable that instantiates or increases with each pass of thefor
loop, rather than using.count()
.
Your code may work in other environments, but for these Treehouse tests they're looking for very specific things, so it pays to slow down and spell things out explicitly. I hope this helps! Please mark this as the Best Answer if you found it most helpful.
Thanks and Be Well, Graham
Srikanth Srinivas
1,465 PointsSrikanth Srinivas
1,465 Pointswhat about this code? it works in theory, but when i enter it on the challenge, it says "wheres word_count()?"
Graham Mackenzie
2,747 PointsGraham Mackenzie
2,747 PointsSo, this is much better along a lot of the lines I was suggesting, but there are still some issues:
You are still assigning
anystring.lower()
to itself. Assign it to a new variable, and then assign the.split()
version of that variable tosplitword
.Where did the variable
x
come from? Did you mean to usecount
?Rather than checking
if word in anystring
, I think you mean to checkif word in any_dict
.Let's go through the logic in your
for
loop: So, we start with a word that is insplitword
.count
gets set equal to 1. Then, we check to seeif word in any_dict
(let's say that it is.) Then (let's say we assume you meant count instead of x)count
gets increased by 1. Then we applycount
(which is now equal to 2) to the entry for thatword
inany_dict
. But we only meant to increase the value by 1. Do you see the problem here? It would be better to setcount = 0
and then use anif... else...
statement to either increase the value ofany_dict[word]
by 1, or else setcount = 1
.I hope this helps!
Be Well, Graham