Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial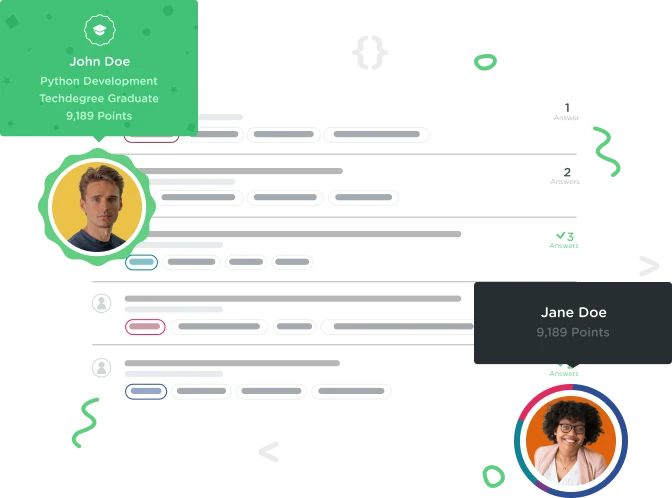

Thomas Verst
725 PointsWhy doesn't this method work?
public class test {
public static void main(String[] args) { String head = System.out.readLine("What character do you want? "); System.out.printf("We are making a new PEZ Dispenser"); System.out.printf("The dispenser is %s", head); } }
4 Answers
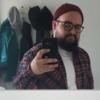
Gabe Olesen
1,606 PointsHi Thomas!
Your code:
public class test {
public static void main(String[] args) {
String head = System.out.readLine("What character do you want? ");
System.out.printf("We are making a new PEZ Dispenser");
System.out.printf("The dispenser is %s", head);
}
}
The line that's having issues is:
String head = System.out.readLine("What character do you want? ");
I assume in your code you've imported one of the below:
import java.io.Console;
import java.util.Scanner;
For the above line to work and call the "readLine" or "nextLine" method on the Console or Scanner class you will need to do the following:
import java.io.Console;
public class test {
public static void main(String[] args) {
Console console = System.console()
String head = console.readLine("What character do you want? ");
}
}
The above will print "What character do you want" on the command line then prompt the user to enter in the character.
import java.util.Scanner;
public class test {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in)
System.out.print("What character do you want? ");
String head = scanner.nextLine();
}
}
I hope this makes sense!
~Gabe
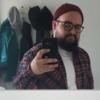
Gabe Olesen
1,606 PointsNo problem!
If you look just below where you type an answer you'll see:
"Reference this Markdown Cheatsheet for syntax examples for formatting your post."
Click onto: Markdown Cheatsheet for the syntax
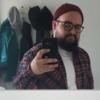
Gabe Olesen
1,606 PointsHey Thomas,
You don't need to use the Scanner, it's just another way of obtaining user input, that's why I gave both examples.
Hope this helped!

Nikola Vichev
1,668 PointsI'm writing the code exactly as it is in the video (at least I think so) and it just won't work ...help, please
public class Example {
public static void main(String[] args) { System.out.println("We are making a new PEZ Dispenser"); PezDispenser dispenser = new PezDispenser("Yoda");
System.out.printf("The dispenser is %s %n",
dispenser.getCharacterName()
);
String before = dispenser.swapHead("Darth Vader");
System.out.printf("It was %s but Chris switched it to %s %n,
before,
dispenser.getCharacterName());
}
}
...and I'm getting
Example.java:13: error: ';' expected
dispenser.getCharacterName()
^
Example.java:13: error: ';' expected
dispenser.getCharacterName()
^
Thomas Verst
725 PointsThomas Verst
725 PointsThanks!! It was very helpful!! Just one question, how did you send me the code like that? I mean, with the dark background, colors and stuff...?
Thomas Verst
725 PointsThomas Verst
725 PointsFor what do I need the scanner actually? My code works totally fine without it...