Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial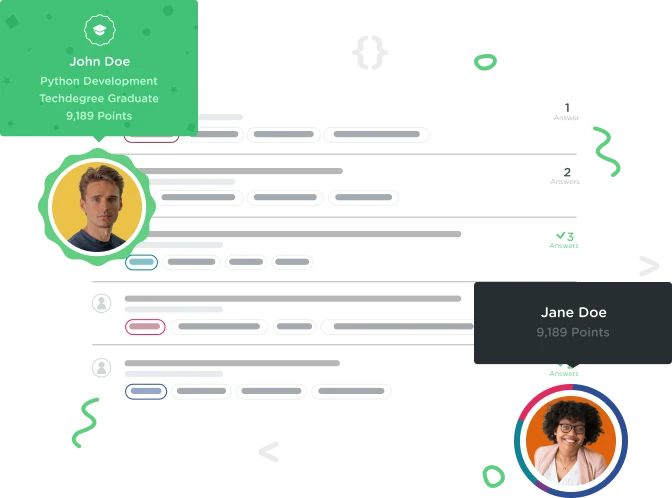

Jackson Monk
4,527 PointsWhy doesn't this work?
I am working in Notepad++ and everything shows up on the page well, but the page doesn't seem to be reading the Javascript I put between the script tags, and I'm not sure why. Code is below, help is appreciated.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<style>
.home {
position: absolute;
padding: 15px;
left: 475px;
top: 0;
right: 325px;
border-bottom-left-radius: 5px;
border-top-left-radius: 5px;
font-size: 15px;
background-color: transparent;
border: 0;
color: black;
}
.about {
position: absolute;
padding: 15px;
left: 546px;
top: 0;
font-size: 15px;
background-color: transparent;
border: 0;
color: black;
}
.instruments {
position: absolute;
padding: 15px;
left: 616px;
top: 0;
font-size: 15px;
background-color: transparent;
border: 0;
color: black;
}
.meet {
position: absolute;
padding: 15px;
right: 508px;
top: 0;
font-size: 15px;
border-bottom-right-radius: 5px;
border-top-right-radius: 5px;
border-left: -10px;
background-color: transparent;
border: 0;
color: black;
}
.decal {
position: absolute;
width: 100%;
height: 80%;
top: 0px;
left: 5px;
right: 5px;
border-radius: 10px;
filter: opacity(35%);
}
.title {
position: absolute;
top: 210px;
left: 500px;
font-family: Impact, fantasy;
font-size: 50px;
}
</style>
</head>
<body>
<script>
window.onload = function() {
let buttons = document.getElementsByTagName('button');
buttons[0].addEventListener('mouseout', () => {
buttons[0].style.backgroundColor = 'transparent';
buttons[0].style.color = 'black';
});
buttons[0].addEventListener('mouseout', () => {
buttons[0].style.backgroundColor = 'transparent';
buttons[0].style.color = 'black';
});
};
</script>
<div>
<button class = home>Home</button>
<button class = about>About</button>
<button class = instruments>Instruments</button>
<button class = meet>Meet The Band</button>
</div>
<img class = decal src = http://www.rockguitarlessons.net/wp-content/uploads/2011/01/rock_guitar.jpg>
<h1 class = title align = center>EXTERMINATION</h1>
</body>
</html>
3 Answers

Jackson Monk
4,527 PointsI have tried your suggestion, but it doesn't work in Notepad. However it does work in other text editors I've tried, which leads me to believe that it is just a problem with notepad

Jackson Monk
4,527 PointsI have tried using other JavaScript methods in Notepad and none of them work. Something has to be wrong with how the JavaScript is loading

Jackson Monk
4,527 PointsI'm so stupid, I was accidentally writing the css you told me in the script tags lol. Sorry, your code works perfect. Thank you!

Vincent Kempers
4,942 PointsNo Problem, glad to help!
Vincent Kempers
4,942 PointsVincent Kempers
4,942 PointsYour code works! For this fix in your current code i suggest the following:
You are changing the home button (the first in the buttons array) from transparent to transparent. In that case you wouldn't know if it is actually changing. I suggest to see the effect of your code to change the
background-color
of the home button (in your css) to red.After this you can mouseout the button and it would change!
Happy Coding!