Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial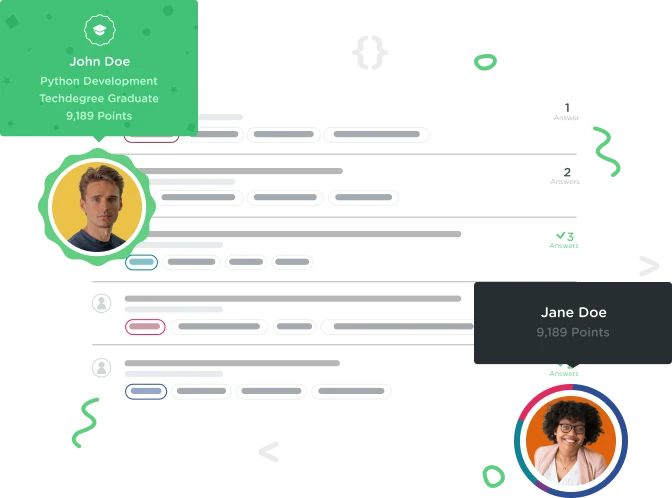
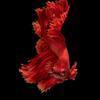
Michael Williams
Courses Plus Student 8,059 PointsWhy don't I need to give my printError function a parameter?
Andrew really whizzed right through the last bit of this video. We changed out all of the console.error
s with our printError
function defined at the top, including arguments. However, on line 40 (I've commented it out and pointing to it with a big arrow to make it obvious which line at the bottom), we didn't give it an argument like we did the others. So I'm a little confused as why.
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
//Require https module
const https = require('https');
// Print Error Messages
function printError(error) {
console.error(error.message);
}
//Function to print message to console
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript`;
console.log(message);
}
function getProfile(username) {
try {
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch (error) {
printError(error);
}
});
});
// request.on('error', error => printError); <---------------------This is what I'm talking about
} catch (error) {
printError(error);
}
}
const users = process.argv.slice(2);
users.forEach(getProfile);
6 Answers
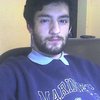
Dario Bahena
10,697 PointsIt is a mistake. It should be:
request.on('error', error => printError(error));
// or this works too because the argument is
// passed automatically to the printError function and run
request.on('error', printError);
Simpler example:
function print(data) {
console.log(data);
}
const foo = [1, 2, 3, 4];
foo.forEach( x => print); // this will not execute and just return undefined
foo.forEach( x => print(x)); // this will print correctly

Jazz Jones
8,535 PointsI haven't taken this course yet, or really did anything with Node.js that didn't have the express framework, but from glancing at it that line of code I think it'll just print undefined in the console, because you're not passing it the error object. Plus it seems pointless to have an on error function with the request object, as you already have the try catch block collecting any and all errors. I'd think it'd be safe just to remove that line.
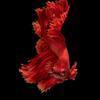
Michael Williams
Courses Plus Student 8,059 PointsYeah, I'm a little confused by it as well, as to why we need it. I'm wondering if it was just to show us how to do it.
Maybe I'm just having a dense moment, but I don't see how it will print the error if you're not passing an argument which it says that it needs to run the function.
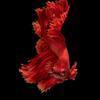
Michael Williams
Courses Plus Student 8,059 PointsThank you, Dario Bahena. The weird thing is that my program still ran. So I'm stumped.
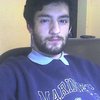
Dario Bahena
10,697 PointsYour program will still work because that piece of code will only run if there is an error that happens on a request. If there are no requests with errors, that function will never run. Further more, if there was an error on a request what will run is just a function that returns a function because the printError function is not actually being called/invoked.
So in the example I gave foo.forEach(x=> print)
This is syntactically correct although logically not. This is why your program still runs.
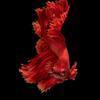
Michael Williams
Courses Plus Student 8,059 PointsAhhhhhhh. Thanks, Dario Bahena. I undestand 100% now.
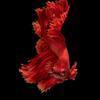
Michael Williams
Courses Plus Student 8,059 Pointsyoav green Can you explain? I don't fully understand your question.
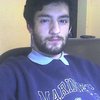
Dario Bahena
10,697 Points@yoav green
This is what is happening under the hood. In essence, the print function is given as a parameter. The anything function then runs it if its condition is met. In JavaScript, functions can be passed as regular arguments. In other words, just because it is a function, does not mean it has to be invoked directly.
In the anything function, fn is a variable that references whatever value you pass it. The way this function is written, when you give fn the value of a function, it will call it as a function.
function print(data) {
console.log(data);
}
function anything(condition, fn) {
const str = "some string";
if (condition) fn(str);
}
anything(true, print);
anything(true, whatever => print(whatever));
yoav green
8,611 Pointsyoav green
8,611 Pointswhy the argument is passed automatically?
JASON LEE
17,351 PointsJASON LEE
17,351 PointsI think the original poster is asking about these cases