Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial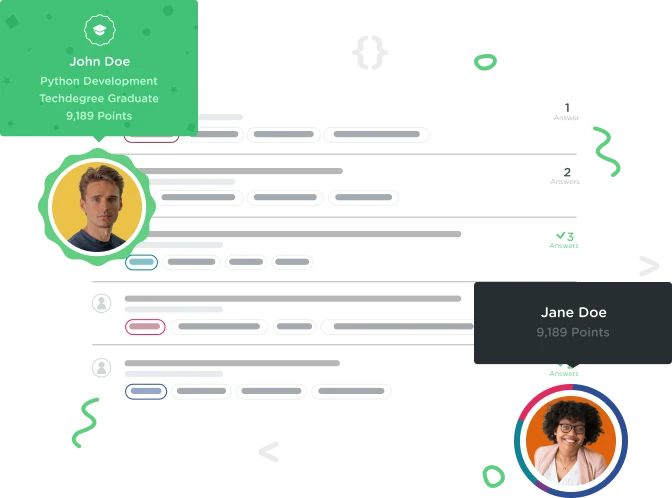
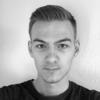
Marcus Klausen
17,425 PointsWhy don't I need to override the init method?
I've made my own code and examples for better understanding. However in my own code examples the OVERRIDE keyword is not needed.. why? Could someone please specify the override function what it does and when it's needed and so own?
//: Playground - noun: a place where people can play
import UIKit
// The model of a basic weapon.
class Weapon {
let name: String
var range: Int
let damage: Int
init(name: String, range: Int, damage: Int) {
self.name = name
self.range = range
self.damage = damage
}
}
// The model of a basic melee weapon
class Melee: Weapon {
init(name: String, damage: Int) {
super.init(name: name, range: 1, damage: damage)
}
}
// The model of area-of-effect / grenade weapons
class Grenade: Weapon {
var area: Int
init(name: String, range: Int, damage: Int, area: Int) {
self.area = area
super.init(name: name, range: range, damage: damage)
}
}
// making an instance of a basic weapon
var basicGun = Weapon(name: "AK-47", range: 30, damage: 20)
// making an instance of a basic melee weapon
var basicSword = Melee(name: "Great Sword", damage: 40)
// making an instance of a basic grenade
var basicGrenade = Grenade(name: "Explosive Grenade", range: 5, damage: 25, area: 40)
// Testing the properties
basicGun
basicGun.name
basicGun.range
basicGun.damage
basicSword
basicSword.name
basicSword.range
basicSword.damage
basicGrenade
basicGrenade.name
basicGrenade.damage
basicGrenade.range
basicGrenade.area
1 Answer
Rodrigo Chousal
16,009 PointsHey Marcus,
According to the documentation on overriding designated initialisers, "When you write a subclass initializer that matches a superclass designated initializer, you are effectively providing an override of that designated initializer". What this means is you only write the override modifier when your subclass is initialising the same properties, but differently than the superclass designated initialiser. But because in your playground you are merely adding one more property but initialising the rest the same way as the superclass, the override modifier isn't needed.
Hope this helps, Rodrigo
Marcus Klausen
17,425 PointsMarcus Klausen
17,425 PointsRead your answer and watched the video again.. all makes sense now.. thank you, much appreciated! :-)