Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial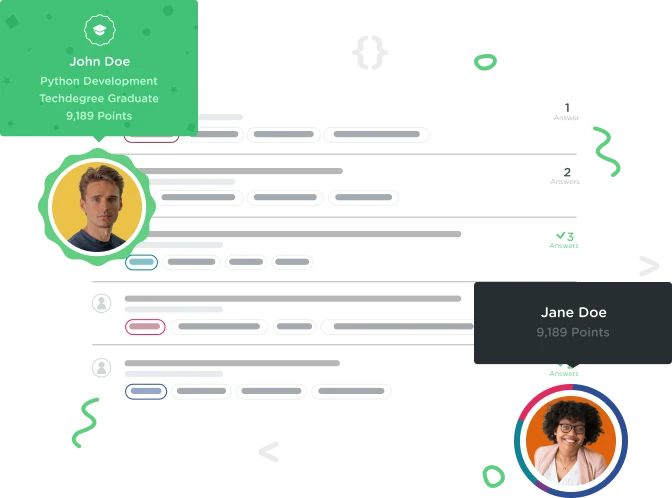

mattcleary2
19,953 PointsWhy don't I see anything until after I quit?
Hey guys,
I'm trying to follow along in my workspace.
For some reason, my code more or less works yet I don't see anything on the screen, aside from the title, until AFTER I've quit.
Then all my inputs and outputs suddenly appear.
Can you please help me out?
This is my code:
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while(true){
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
search = search.toLowerCase();
if(search === 'quit'){
break;
}else if (search === 'list') {
print(inStock.join(', '));
}else{
if(inStock.indexOf(search) > -1){
print('Yes, we have ' + search + ' in the store.');
} else {
print(search + ' is not in stock.');
}
}
}
4 Answers
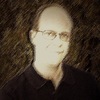
Jason Anders
Treehouse Moderator 145,860 PointsHey Matt,
Are you talking about quitting Workspaces or Treehouse.
Changes you make in Workspaces, do NOT auto-refresh in the browser. So, after every change, you will need to refresh the browser window, and sometimes even clear the cache.
Personally, I don't use Workspaces, but my suggestion is after every 'view,' close the tab the preview is in, and this way when you re-open a preview, it is (usually) a fresh one.
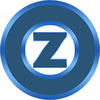
Daniel Gauthier
15,000 PointsHey Matt,
The way you've constructed your if statement is causing the loop to continue iterating, even after a decision other than quit is made.
By adding break statements to each of the decisions, the loop will stop and the print statements will show as desired. If you wanted the prompt to reappear, you would have to trigger it again with another line of code, but the code below will solve the current problem and prevent the behavior you're having trouble with at the moment.
Here's the modified code I used:
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while(true){
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
search = search.toLowerCase();
if(search === 'quit'){
break;
}else if (search === 'list') {
print(inStock.join(', '));
break;
}else{
if(inStock.indexOf(search) > -1){
print('Yes, we have ' + search + ' in the store.');
break;
} else {
print(search + ' is not in stock.');
break;
}
}
}
Good luck with the course!

Valerio Viperino
6,947 PointsWhile this could solve the question (we just need a button click for entering the "search engine"), the instructor's browser actually seems to immediately evaluate the document.write() statement before the new loop begins. Maybe he can shed some light on this.
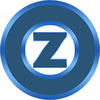
Daniel Gauthier
15,000 PointsHey Valerio,
Manav Misra responded to a question similar to this one recently and provided a correct answer about the browser being the culprit.
Firefox will behave the way Dave's example does in the video, while Chrome will wait to print until the prompts are interrupted.
There are ways around this, but that's a topic for another time :D
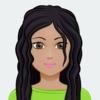
Jeeya M
16,839 PointsIf you have this problem again you can go to the console and usually it will say where the problem is.
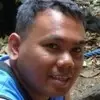
Dennis Amiel Domingo
17,813 PointsWhen you open the Workspace for this video, you'll notice that Dave posted a comment about this problem:
"/* Important note The behavior of most browsers has changed since this video was shot, so you won't see the same thing as I demonstrate in the video. In the video, you'll see that my script is able to print out to the browser using document.write( ) while inside a loop.
Most browsers no longer do that: they wait until the loop finishes and then they print to the window. So, you'll see a blank page until you type quit in the prompt window — then you'll see all the output printed to the screen.
Sorry for the confusion, and we'll update the video soon. */"
Now, to solve this problem, replace all instances of "print" with "alert" and you'll see your list as well as the other messages.
Example:
From
print( instock.join(', '));
To
alert( instock.join(', '));