Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial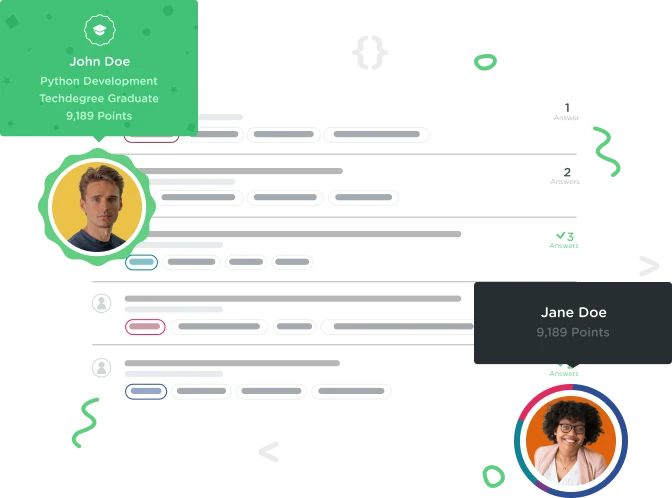

Greg Schudel
4,090 PointsWhy element is not being removed??
Here is my HTML:
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>RSVP App</title>
<link href="https://fonts.googleapis.com/css?family=Courgette" rel="stylesheet">
<link href="https://fonts.googleapis.com/css?family=Lato:400,700" rel="stylesheet">
<link href="css/style.css" rel="stylesheet">
</head>
<body>
<div class="wrapper">
<header>
<h1>RSVP</h1>
<p>A Treehouse App</p>
<form id="registrar">
<input type="text" name="name" placeholder="Invite Someone">
<button type="submit" name="submit" value="submit">Submit</button>
</form>
</header>
<div class="main">
<h2>Invitees</h2>
<ul id="invitedList"></ul>
</div>
</div>
</body>
<script src="app.js"></script>
</html>
Here is my JS
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const ul = document.getElementById('invitedList'); // creates element ul
function createLi (text) {
const li = document.createElement('li'); // creates an li element forom the input
li.textContent = text; // changes the element to text
const label = document.createElement('label'); //creates a label element
label.textContent = 'Confirmed'; // changes the text to say "confirmed"
const checkbox = document.createElement('input'); //creates an input element
checkbox.type = 'checkbox'; // changes the input element ot a checkbox element
label.appendChild(checkbox); // appends the label to the front of the checkbox
li.appendChild(label); //appends the label within the li element
const editButton = document.createElement('button'); //creates a button element
editButton.textContent = 'Edit'; //makes the button element to say remove
li.appendChild(editButton); // appends the button within the li element
const removeButton = document.createElement('button'); //creates a button element
removeButton.textContent = 'Remove'; //makes the button element to say remove
li.appendChild(removeButton); // appends the button within the li element
return li; // returns the li through the conveyer
}
form.addEventListener('submit', (e) => {
e.preventDefault(); // prevents browsers default action
const text= input.value; //grabs the value from the user
input.value = ''; // clears the value after submission
const li = createLi(text); // this grabs the input and "javascript conveyer belts" it through the createLi function
ul.appendChild(li); // grabs the li outputed from the above modular function and then appends to the DOM
});
ul.addEventListener('change' , (e) => {
const checkbox = event.target; // references to checkbox itself
const checked = checkbox.checked; // stores value of checkbox (true/checked or false/unchecked)
const listItem = checkbox.parentNode.parentNode; // travarses two elements
if (checked){
listItem.className = 'responded';
}
else{
listItem.className = '';
}
});
ul.addEventListener('click' , (e) => {
if (e.target.tagName === "BUTTON") { // button in all caps cause tagName value REQUIRES THIS
const buttonPress = e.target;
const li = buttonPress.parentNode;
const ul = li.parentNode;
if ( buttonPress.textContent === "Remove" ) {
ul.removeChild(li);
} else if ( buttonPress.textContent === "Edit" ) {
const span = li.firstElementChild; // selecting the text that is not a span
const input = document.createElement('input'); // creating an element for user input
input.type = 'text' // this tells the input element that I want it to accept text
li.insertBefore(input, span); // while referencing the li, which traverses the DOM from the base point of the button element, you use the 'insertBefore' property to insert the new input element before the span element
li.removeChild(span);
buttonPress.textContent = 'Save';
}
}
});
I know the answer is in the video, but I am struggling to troubleshoot this by myself so I can learn this. Why is it when I hit the dit button, the input box comes up but the span element that has the name is not being removed by the removeChild
method?? What am I doing wrong???
3 Answers
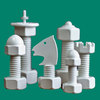
Steven Parker
231,275 PointsThe comments don't match what the code is doing, which makes things a bit more confusing, for example:
const span = li.firstElementChild; // selecting the text that is not a span
There are no "span" elements in the li; and the first element is the label (and the checkbox), which is what this selects.
The "removeChild" then successfully removes the label and checkbox.

Greg Schudel
4,090 PointsThere was another modular function that I forgot I needed to change
function createLi (text) {
const li = document.createElement('li');
// I had to add the below three lines
const span = document.createElement('span');
span.textContent = text;
li.appendChild(span);
const label = document.createElement('label');
label.textContent = 'Confirmed';
const checkbox = document.createElement('input'); //creates an input element
checkbox.type = 'checkbox'; // changes the input element ot a checkbox element
label.appendChild(checkbox); // appends the label to the front of the checkbox
li.appendChild(label); //appends the label within the li element
const editButton = document.createElement('button'); //creates a button element
editButton.textContent = 'Edit'; //makes the button element to say remove
li.appendChild(editButton); // appends the button within the li element
const removeButton = document.createElement('button'); //creates a button element
removeButton.textContent = 'Remove'; //makes the button element to say remove
li.appendChild(removeButton); // appends the button within the li element
return li; // returns the li through the JS "conveyer belt"
}
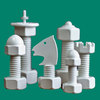
Steven Parker
231,275 PointsSo did that fix it, or do you still need assistance?
And if the latter, is this in a workspace where you could make a snapshot and post the link to it here?

Greg Schudel
4,090 Pointsthat fixed it, I had to create the element and append it first before I could get it to work properly. Basically I was trying to do something all in one function rather then spread it out like the teacher did.
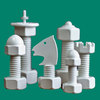
Steven Parker
231,275 PointsGood deal, and it explains why that label was put in a variable named "span".