Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial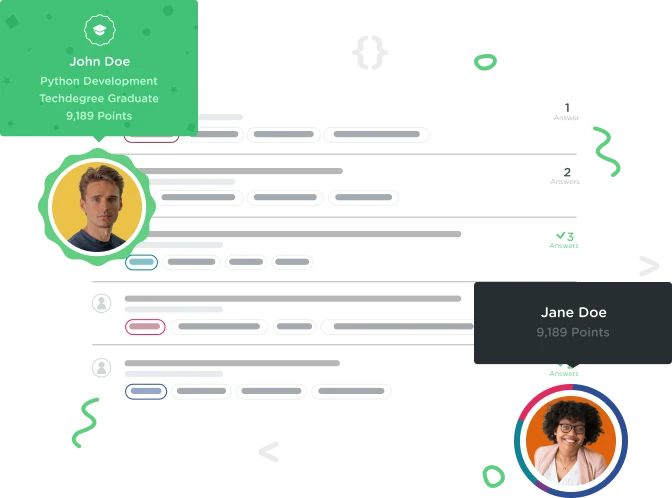
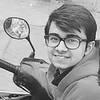
Kanish Chhabra
2,151 PointsWhy enums over objects?
We could've used structures to perform the exact same task for providing RGB and HSB values, why did we use enums then?
3 Answers
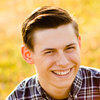
Caleb Kleveter
Treehouse Moderator 37,862 PointsEnums integrate deeply with switch
statements. They also have a 'feel' to them that after you have been using Swift for awhile makes them seem beautiful. Another use for enums is error handling. This gets covered in a later course.
Maybe to help explain the reason for enums, I will show you some example code from a side project I am working on. It is an enum that works as a wrapper around a font set like IonIcons, but for weather, called Weather Icons.
enum Icon {
case clearDay
case clearNight
case rain
case snow
case sleet
case wind
case fog
case cloudy
case partlyCloudyDay
case partlyCloudyNight
case unSupported
case up
case down
case humidity
case precipitation
var hex: String {
switch self {
case .clearDay: return "\u{f00d}"
case .clearNight: return "\u{f02e}"
case .rain: return "\u{f019}"
case .snow: return "\u{f01b}"
case .sleet: return "\u{f0b5}"
case .wind: return "\u{f050}"
case .fog: return "\u{f014}"
case .cloudy: return "\u{f013}"
case .partlyCloudyDay: return "\u{f002}"
case .partlyCloudyNight: return "\u{f086}"
case .unSupported: return "\u{f07b}"
case .up: return "\u{f058}"
case .down: return "\u{f044}"
case .humidity: return "\u{f07a}"
case .precipitation: return "\u{f04e}"
}
}
static func getIcon(from string: String) -> Icon {
switch string.uppercased() {
case "CLEAR-DAY": return .clearDay
case "CLEAR-NIGHT": return .clearNight
case "RAIN": return .rain
case "SNOW": return .snow
case "SLEET": return .sleet
case "WIND": return .wind
case "FOG": return .fog
case "CLOUDY": return .cloudy
case "PARTLY-CLOUDY-DAY": return .partlyCloudyDay
case "PARTLY-CLOUDY-NIGHT": return . partlyCloudyNight
default: return .unSupported
}
}
}
In this case, an enum makes a lot more sense then a Struct with static vars. When you have a Struct that works like an enum, you end up using the Struct wrong, That is what enums are designed for.
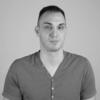
eberhapa
51,495 PointsAn enum is a struct under the hood. Its looks a little bit better for such small tasks where you have fixed values to choose from. If you would do it as a struct you would have to instantiate the struct or do static properties and create every single value yourself. An enum does that for you.
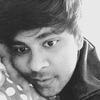
SivaKumar Kataru
2,386 PointsIt is used to organise a finite set of data.
akyya mayberry
3,668 Pointsakyya mayberry
3,668 PointsCan you maybe post how you would do the struct implementation of this enum?